In this post we shall learn on how to create a new shipping method in addition to existing ones and exploring some options from Shipping Method API of WooCommerce. Since version 2.6 of WooCommerce, there are only 3 default types of shipping methods. They are as follows:
- Flat Rate
- Free Shipping and
- Local Pickup
Let us follow the below mentioned steps to add our new shipping method called Tyche Shipping.
Where to Add Custom Code in WooCommerce?
It is advisable to add the code snippets to the functions.php file of your child theme. Access the file directly from Appearance->Theme File Editor->Locating the child theme’s functions.php from the right sidebar. You can also access it from your theme’s directory file. Insert the following code snippet in functions.php. The alternative & easy option is to install & activate the Code Snippets plugin. You can then add the code as a new snippet via the plugin.
1. Register shipping method
WooCommerce has a filter called woocommerce_shipping_methods
. The function hooked to this filter will have the current available shipping methods in the form of an array. We shall add our shipping method tyche_method as shown in the below snippet.
Note that WC_Shipping_Tyche is the name of the class for which we are adding the shipping method. Here is a sample snippet of the class WC_Shipping_Tyche which is an extension of WC_Shipping_Method class.
add_filter( 'woocommerce_shipping_methods', 'register_tyche_method' ); function register_tyche_method( $methods ) { // $method contains available shipping methods $methods[ 'tyche_method' ] = 'WC_Shipping_Tyche'; return $methods; } /** * WC_Shipping_Tyche class. * * @class WC_Shipping_Tyche * @version 1.0.0 * @package Shipping-for-WooCommerce/Classes * @category Class * @author Tyche Softwares */ class WC_Shipping_Tyche extends WC_Shipping_Method { /** * Constructor. The instance ID is passed to this. */ public function __construct( $instance_id = 0 ) { $this->id = 'tyche_method'; $this->instance_id = absint( $instance_id ); $this->method_title = __( 'Tyche Shipping Method' ); $this->method_description = __( 'Tyche Shipping method for demonstration purposes.' ); $this->supports = array( 'shipping-zones', 'instance-settings', ); $this->instance_form_fields = array( 'enabled' => array( 'title' => __( 'Enable/Disable' ), 'type' => 'checkbox', 'label' => __( 'Enable this shipping method' ), 'default' => 'yes', ), 'title' => array( 'title' => __( 'Method Title' ), 'type' => 'text', 'description' => __( 'This controls the title which the user sees during checkout.' ), 'default' => __( 'Tyche Shipping Method' ), 'desc_tip' => true ) ); $this->enabled = $this->get_option( 'enabled' ); $this->title = $this->get_option( 'title' ); add_action( 'woocommerce_update_options_shipping_' . $this->id, array( $this, 'process_admin_options' ) ); } /** * calculate_shipping function. * @param array $package (default: array()) */ public function calculate_shipping( $package = array() ) { $this->add_rate( array( 'id' => $this->id . $this->instance_id, 'label' => $this->title, 'cost' => 100, ) ); } }
Let’s take a look at a few properties of the WC_Shipping_Method that we just extended to create a new class for our Tyche Shipping Method.
- id – A unique text based ID for the new shipping method you are adding. Here we have used our ID as
tyche_method
. - instance_id – Unique instance ID of the method (zones can contain multiple instances of a single shipping method). This ID is stored in the database.
- method_title – The title applied to the shipping method. This is not the same title that will be displayed on the front-end on checkout page but used only for backend settings. For our shipping method we have used Tyche Shipping Method as the title
- supports – This property is used to determine which all features the new shipping method will support. This property is an array with possible values as:
- shipping-zones – Shipping zone functionality.
- instance-settings – Instance settings screens.
- settings – Non-instance settings screens. Enabled by default for backward compatibility with methods before instances existed.
- instance-settings-modal – Allows the instance settings to be loaded within a modal in the zones UI.
- enabled – Stores whether or not the instance or method is enabled. In the above snippet we have assigned the instance which is saved in database. This value will be displayed in Shipping Methods section of the shipping zone.
- title – Stores the title of your method which can be used on the frontend for Checkout and Cart Pages.
- instance_form_fields – This property is an array of settings fields used at instance level. Here we have shown just one setting which is the title to be displayed on the frontend of the store that we had discussed above.
Now that the shipping method has been registered, let us verify and check if it is getting displayed and settings getting saved and applied.
2. Verify the newly created shipping method
The settings for shipping are available under WooCommerce -> Settings -> Shipping. Let us create a new Shipping Zone by clicking on Add Shipping Zone.
On clicking on Add Shipping Method under Shipping Methods tab, a pop-up modal shall be displayed like one shown below. Now you will notice our newly created shipping method Tyche Shipping Method getting listed in the dropdown list.
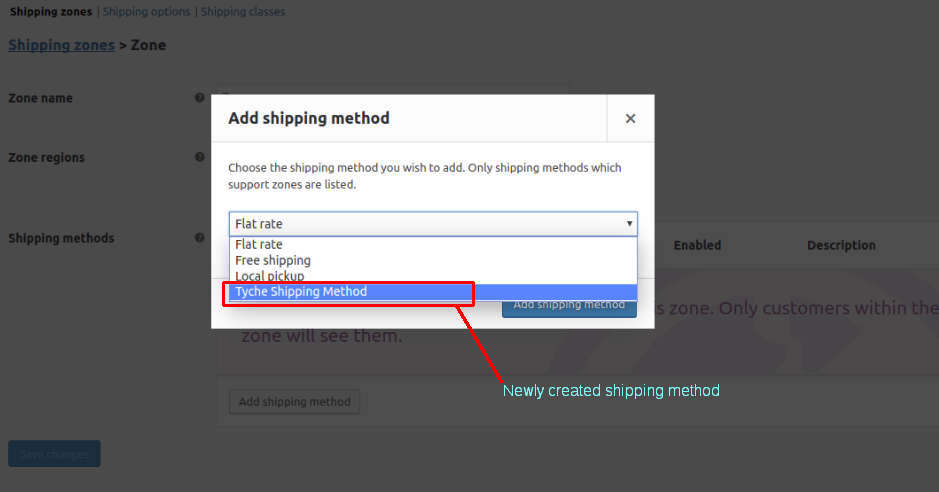
The settings associated with this shipping method, in our case the Title to be displayed on frontend can be configured and edited as per our needs. Just a reminder that we had added title element in instance_form_fields property of the Shipping Method API.
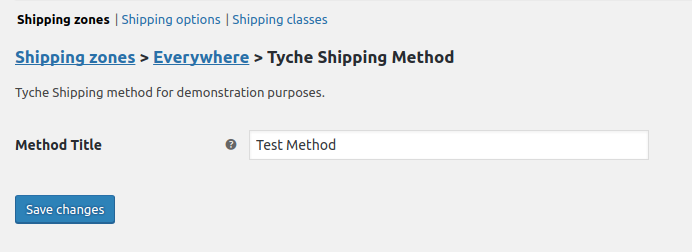
The above setting can also be displayed in a modal pop-up by adding instance-settings-modal
value to supports
property array. The default value, Test Method can be changed by changing the value of the default key of the title
element of instance_form_fields
.
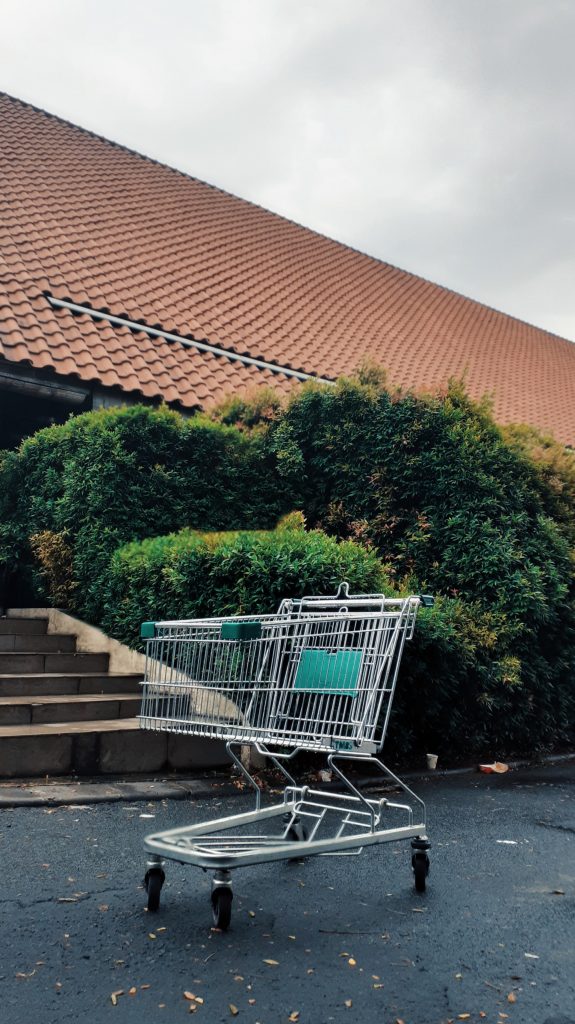
Wondering how to bring back your Abandoned cart users?
Abandoned Cart Pro for WooCommerce is loaded with useful features that help you send an effective volume of reminders via text, email, and messenger. You can also share and manage discount codes that will encourage your customers to complete their orders.
Even better, you can stop the cart abandonment with the exit intent popup!
2. Price calculations for newly created Shipping Methods
If the shipping method is enabled it will be displayed on the Checkout page, but how about the pricing for the Shipping Method? We have a few methods or functions which are provided by the Shipping Method API which we shall use to add pricing options to the shipping method. Let us quickly go through those functions.
- calculate_shipping( package )
This function is called to calculate shipping for a package. The function accepts an array containing the package of items to be shipped. One should use ::add_rate()
to register calculated rates.
- add_rate( args )
This is the actual function that adds rate to the checkout page. It accepts an array as an argument. A brief gist of the args parameter is as follows:
array(
'id' => 'tyche_method',
'label' => 'Tyche Shipping Method',
'cost' => '100',
)
So let us use the above two methods that we just described in our WC_Shipping_Tyche class so that we can add rates on the checkout page. Add the below-given snippet after __construct
the function of WC_Shipping_Tyche class.
4. Verify the Shipping Method on frontend
Now that we also added a rate to our Shipping Method, add a product to your shopping cart and verify the shipping method. You should see something like this:
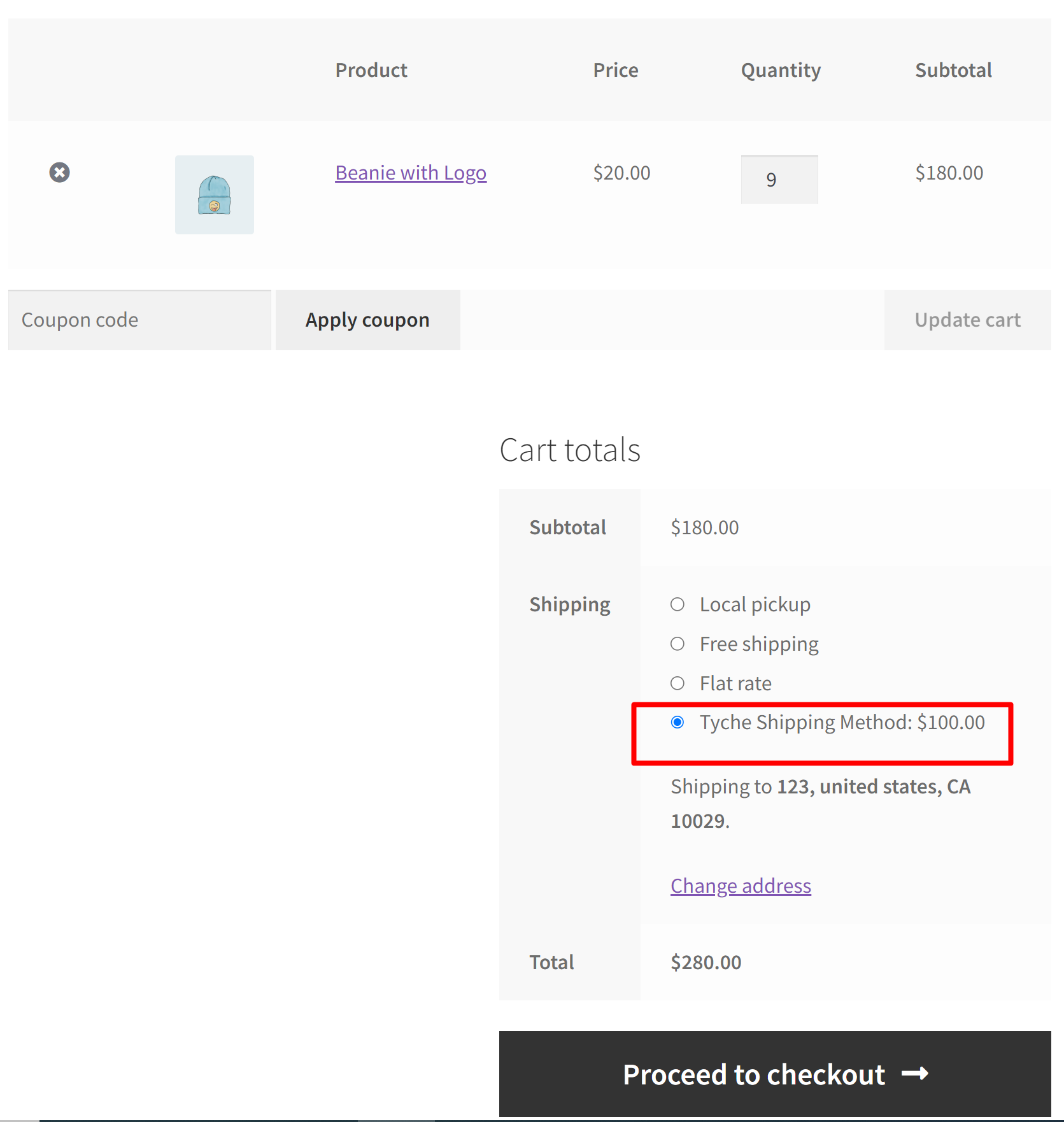
Apart from the properties and methods mentioned above, there are a few other properties and methods as well which can be used to create and tweak a Shipping Method as per your needs. Those are available on the following link.
So after following the above steps, you shall be able to create a new Shipping Method as per your needs and requirements. I hope you find this tutorial useful in creating and modifying Shipping Methods.
Please mention your queries/suggestions/comments in the comments area below and we shall get back to you as early as possible.
how add custom multi origin shipping by product?
Hi Joko, Using the Shipping classes option you can assign shipping methods on per product basis. Firstly, create the shipping classes in the respective shipping zone. And then in the Products tab of your dashboard, Edit each product and assign the appropriate shipping class that matches its shipping origin. This can be done under the ‘Product Data’ -> ‘Shipping’ tab. Please refer to the attached links for more detailed guidance.
https://prnt.sc/QYXVVwaGk7Bt
https://prnt.sc/eqvsReSkcqPE
https://prnt.sc/nEn9auhz_olr
Hi I have a question, its posiible show two cost differentes in the parameter cost?
Hi Sebastian, Certainly! you can show two different costs within the shipping cost parameter in WooCommerce, but it requires some configuration in WooCommerce settings.
Hi thought this code was very useful I found that this repo here is a good example for me to see how to structure these snippets of code https://github.com/woocommerce/woocommerce/wiki/Shipping-Method-API
Hi Marc, I’m glad to hear that you found the code useful! The WooCommerce GitHub repository you’ve shared is indeed a valuable resource for understanding how to structure and implement code snippets related to shipping methods.
When I am processing the order, I have this problem. It keeps loading…
May someone help me?
Hi Tomy, Sorry for the late reply here. We have updated the code & also tested it to work with the most recent version of WooCommerce. Feel free to reply if you have any more issues with it.
You didn’t say where to put all the .php files. Is it under woocommerce plugin? If so, which exact folder? OR do we create a new plugin? If so, it best you tell someone how to create a plugin like what files/folders to create
Great question! The .php files you’re referring to should ideally be placed inside your child theme’s functions.php file for customizing WooCommerce. Also, you can consider using the Code Snippets plugin as an alternative. So that you can create and manage code snippets without modifying core files.