TheBOGO (Buy One, Get One) gift promotion tied to your customers’ delivery date choices is one clever feature that will that will transform your WooCommerce store into a shopping spot! This WooCommerce customization will allow customers to choose a designated day (e.g., Monday) uisng the delivery date field, and a free gift product is automatically added to the cart.
Solution: Add BOGO (Buy One, Get One) Offer for a Specific Delivery Weekday
The below code snippet will add a free gift product to the cart if the chosen delivery date corresponds to a specified day (e.g., Monday).
// Custom function that handles your settings function ts_delivery_date_settings(){ return array( 'targeted_methods' => array('flat_rate:4'), 'field_id' => 'delivery_date', 'field_type' => 'date', 'label_name' => __("Delivery Date","woocommerce"), 'free_gift_day' => 'Monday', // Updated variable name 'free_gift_product_id' => 470, ); } // Display the custom delivery date field add_action( 'woocommerce_after_shipping_rate', 'ts_delivery_date_custom_date_field', 20, 2 ); function ts_delivery_date_custom_date_field( $method, $index ) { extract( ts_delivery_date_settings() ); // Load settings and convert them into variables // Get the chosen shipping methods $chosen = WC()->session->get('chosen_shipping_methods'); $value = WC()->session->get($field_id); $value = WC()->session->__isset($field_id) ? $value : WC()->checkout->get_value('_'.$field_id); if( ! empty($chosen) && $method->id === $chosen[$index] && in_array($method->id, $targeted_methods) ) { echo '<div class="custom-delivery-date">'; woocommerce_form_field( $field_id, array( 'type' => $field_type, 'label' => '', // Not required for date input 'class' => array('form-row-wide ' . $field_id . '-' . $field_type ), 'required' => true, ), $value ); echo '</div>'; } } // Save the delivery date to order add_action('woocommerce_checkout_create_order', 'ts_save_delivery_date_to_order'); function ts_save_delivery_date_to_order($order) { $field_id = 'delivery_date'; if (isset($_POST[$field_id])) { $order->update_meta_data('_'.$field_id, sanitize_text_field($_POST[$field_id])); } } // Display the delivery date in admin orders add_filter('woocommerce_admin_order_data_after_billing_address', 'ts_display_delivery_date_in_admin_orders'); function ts_display_delivery_date_in_admin_orders($order) { $field_id = 'delivery_date'; $value = $order->get_meta('_'.$field_id); if ($value) { echo '<p><strong>'.__('Delivery Date').':</strong> ' . esc_html($value) . '</p>'; } } // jQuery code (client side) - Ajax sender add_action( 'wp_footer', 'ts_delivery_date_script_js' ); function ts_delivery_date_script_js() { // Only cart & checkout pages if( is_cart() || ( is_checkout() && ! is_wc_endpoint_url() ) ): // Load settings and convert them into variables extract( ts_delivery_date_settings() ); $js_variable = is_cart() ? 'wc_cart_params' : 'wc_checkout_params'; // jQuery Ajax code ?> <script type="text/javascript"> jQuery( function($){ if (typeof <?php echo $js_variable; ?> === 'undefined') return false; $(document.body).on( 'change', 'input#<?php echo $field_id; ?>', function(){ var value = $(this).val(); $.ajax({ type: 'POST', url: <?php echo $js_variable; ?>.ajax_url, data: { 'action': 'delivery_date', 'value': value }, success: function (result) { console.log(result); // Only for testing (to be removed) // Check if the selected date is not Monday if (result !== '<?php echo $free_gift_day; ?>') { // Remove the free gift product if the user chooses any other day $.ajax({ type: 'POST', url: <?php echo $js_variable; ?>.ajax_url, data: { 'action': 'remove_free_gift' }, success: function () { // Manually trigger update_checkout $('body').trigger('update_checkout'); // Automatically refresh the cart page window.location.reload(); } }); } else { // Manually trigger update_checkout $('body').trigger('update_checkout'); // Automatically refresh the cart page window.location.reload(); } } }); }); }); </script> <?php endif; } // The WordPress Ajax PHP receiver add_action( 'wp_ajax_delivery_date', 'ts_set_delivery_date' ); add_action( 'wp_ajax_nopriv_delivery_date', 'ts_set_delivery_date' ); function ts_set_delivery_date() { if ( isset($_POST['value']) ){ // Load settings and convert them into variables extract( ts_delivery_date_settings() ); $value = sanitize_text_field( $_POST['value'] ); // Update session variable WC()->session->set( $field_id, $value ); // Check if the selected date is the free gift day (e.g., Monday) if (date('l', strtotime($value)) === $free_gift_day) { // Automatically add the free gift product to the cart WC()->cart->add_to_cart($free_gift_product_id); } else { // Remove the free gift product if the user chooses any other day foreach ( WC()->cart->get_cart() as $cart_item_key => $cart_item ) { if ( $cart_item['product_id'] === $free_gift_product_id ) { WC()->cart->remove_cart_item($cart_item_key); } } // Manually trigger update_checkout WC()->cart->calculate_totals(); } } echo $value; die(); }
Output
When the customer chooses a delivery date that is associated with the weekday ‘Monday’ then the gift is automatically added to the cart.
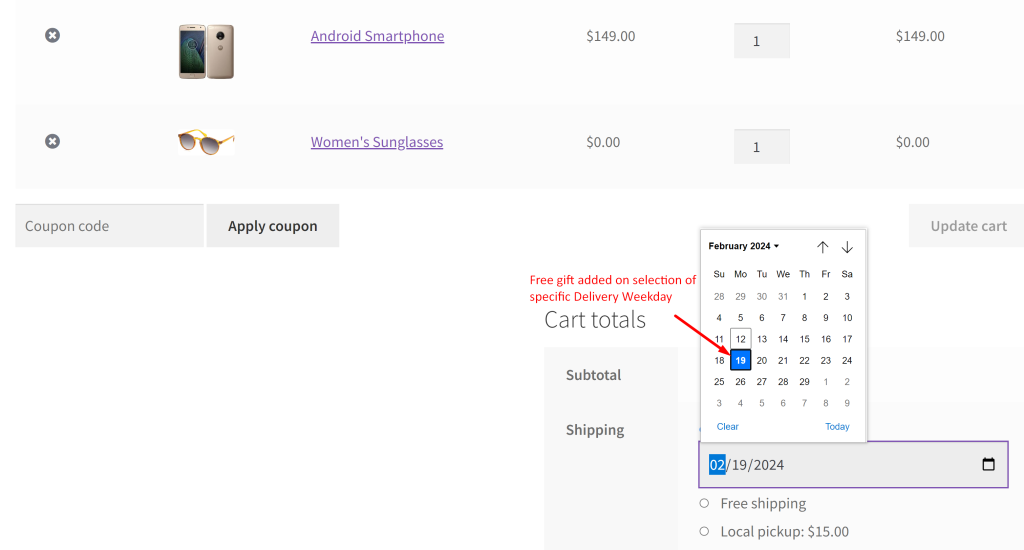
On selection of any other delivery date that is not associated with the weekday ‘Monday’ in such cases, the free product is removed from the cart.
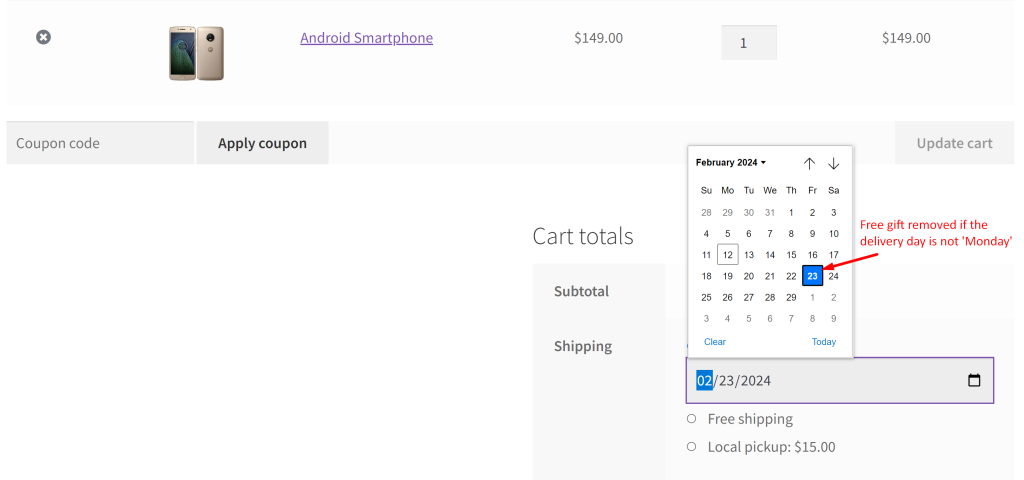
If you want to let your customers choose specific delivery dates, our Order Delivery Date Pro Plugin is the perfect solution. It shows available dates for delivery and lets you set up various options like delivery time slots, holiday schedules, and next-day delivery charges. With this plugin, you can make your store more flexible and better meet your customers’ needs.