Table of Contents
As a store owner, if you ship products at a global level having a delivery partner with a single shipping carrier is not enough. You need to integrate with multiple shipping carriers such as FedEx, UPS, DHL, and DTDC to reach out to more customers. So, providing multiple shipping carrier choices and their associated shipping costs helps customers to choose a reliable carrier they would prefer based on their location, speed, cost, and safety.
This post helps you to add an extra carrier field for shipping methods in WooCommerce cart and checkout page.
Where to Add Custom Code in WooCommerce?
It is advisable to add the code snippets to the functions.php file of your child theme. Access the file directly from Appearance->Theme File Editor->Locating the child theme’s functions.php from the right sidebar. You can also access it from your theme’s directory file. Insert the following code snippet in functions.php. The alternative & easy option is to install & activate the Code Snippets plugin. You can then add the code as a new snippet via the plugin.
Preliminary Steps
- Decide the shipping carriers you wish to place in the carrier fields and the rates that you wish to assign to each carrier.
- Choose the target shipping method. As the rates of the shipping carriers are assigned to the flat rate, make sure to find the flat rate ID. You can do this by going to the WooCommerce checkout page, then right-click on the Shipping method->Select Inspect->Developer tools. e.g. The Flat rate shipping method ID, in this case, is flat_rate: 25.
Solution: Add Carrier Field for Shipping Methods in WooCommerce Cart and Checkout
If your store operates on the global level definitely it might offer different shipping carrier options. Let’s consider that we are adding these shipping carriers FedEx, UPS, DHL, and DTDC on the cart and checkout page. Also, we have set unique rates for these carriers like this:
- FedEx => $15.00
- UPS => $12.00
- DHL=> $18.00
- DTDC => $10.00
The code below will help to offer different shipping carrier options and dynamically calculate the shipping cost based on the selected carrier.
// Custom function that handles your settings function ts_carrier_settings() { return array( 'targeted_methods' => array('flat_rate:25'), // Your targeted shipping method(s) in this array 'field_id' => 'carrier_name', // Field Id 'field_type' => 'select', // Field type 'label_name' => __("Carrier company", "woocommerce"), // Label for validation and as meta key for orders 'field_options' => array( // The carrier companies below (one per line) 'FedEx' => 15.00, // Replace with the flat rate values for each carrier 'UPS' => 12.00, 'DHL' => 18.00, 'DTDC' => 10.00, ), ); } // Display the custom checkout field add_action('woocommerce_after_shipping_rate', 'ts_carrier_company_select_field', 20, 2); function ts_carrier_company_select_field($method, $index) { extract(ts_carrier_settings()); // Load settings and convert them into variables $chosen = WC()->session->get('chosen_shipping_methods'); // The chosen methods $value = WC()->session->get($field_id); $value = WC()->session->__isset($field_id) ? $value : WC()->checkout->get_value('_' . $field_id); $options = array(); // Initializing if (!empty($chosen) && $method->id === $chosen[$index] && in_array($method->id, $targeted_methods)) { echo '<div class="custom-carrier">'; // Add the default option $options[''] = __("Choose a carrier company", "woocommerce"); // Loop through field options to add the correct carrier names foreach ($field_options as $key => $option_value) { if ($key !== 0) { $options[$key] = $key; // Use the carrier name as the label } } woocommerce_form_field($field_id, array( 'type' => $field_type, 'label' => $label_name, // Set the label to "Carrier company" 'class' => array('form-row-wide ' . $field_id . '-' . $field_type), 'required' => true, 'options' => $options, ), $value); echo '</div>'; } } // jQuery code (client-side) - Ajax sender add_action('wp_footer', 'ts_carrier_company_script_js'); function ts_carrier_company_script_js() { // Only cart & checkout pages if (is_cart() || (is_checkout() && !is_wc_endpoint_url())): // Load settings and convert them into variables extract(ts_carrier_settings()); $js_variable = is_cart() ? 'wc_cart_params' : 'wc_checkout_params'; // jQuery Ajax code ?> <script type="text/javascript"> jQuery(function ($) { if (typeof <?php echo $js_variable; ?> === 'undefined') return false; $(document.body).on('change', 'select#<?php echo $field_id; ?>', function () { var value = $(this).val(); $.ajax({ type: 'POST', url: <?php echo $js_variable; ?>.ajax_url, data: { 'action': 'update_shipping_rates', 'value': value }, success: function (result) { // Parse the JSON response var response = JSON.parse(result); if (response.new_rate !== undefined) { // Update the displayed shipping cost $('.woocommerce-shipping-totals .woocommerce-Price-amount').html(response.new_rate); // Update the cart total $('.order-total .woocommerce-Price-amount').html(response.cart_total); } } }); }); }); </script> <?php endif; } // The WordPress Ajax PHP receiver add_action('wp_ajax_update_shipping_rates', 'ts_update_shipping_rates'); add_action('wp_ajax_nopriv_update_shipping_rates', 'ts_update_shipping_rates'); function ts_update_shipping_rates() { if (isset($_POST['value'])) { // Load settings and convert them into variables extract(ts_carrier_settings()); $selected_carrier = esc_attr($_POST['value']); // Get the flat rate value for the selected carrier $new_rate = isset($field_options[$selected_carrier]) ? $field_options[$selected_carrier] : 0; // Get the product subtotal $product_subtotal = WC()->cart->get_cart_contents_total(); // Calculate the new cart total by adding the product subtotal and the new shipping rate $new_cart_total = $product_subtotal + $new_rate; // Set the cart total to the new calculated total WC()->cart->set_total($new_cart_total); // Send back the new cart total to JavaScript (json encoded) echo json_encode(array('new_rate' => wc_price($new_rate), 'cart_total' => wc_price($new_cart_total))); die(); } }
Output
Use case: List out carrier options in the drop-down field
When a customer selects the ‘flat rate’ shipping option the shipping carrier fields will be displayed with a list of shipping carrier options. The below output shows that the fields are displayed both on the cart and checkout page.
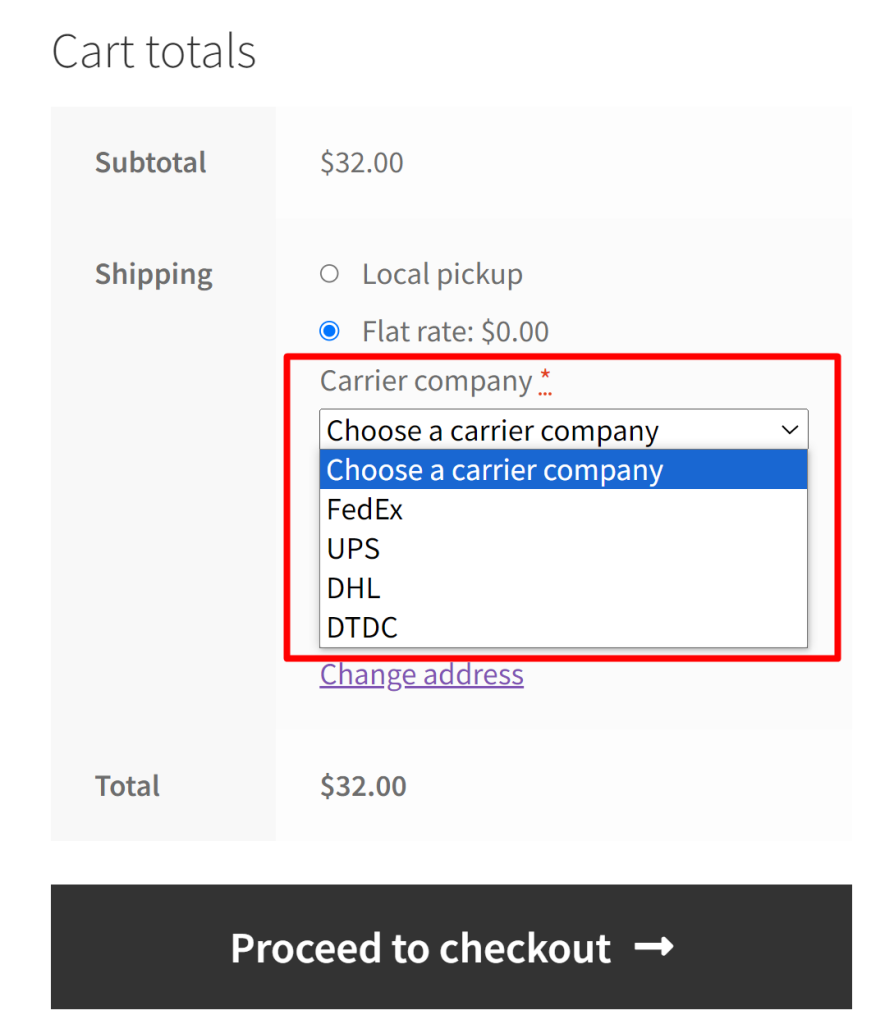
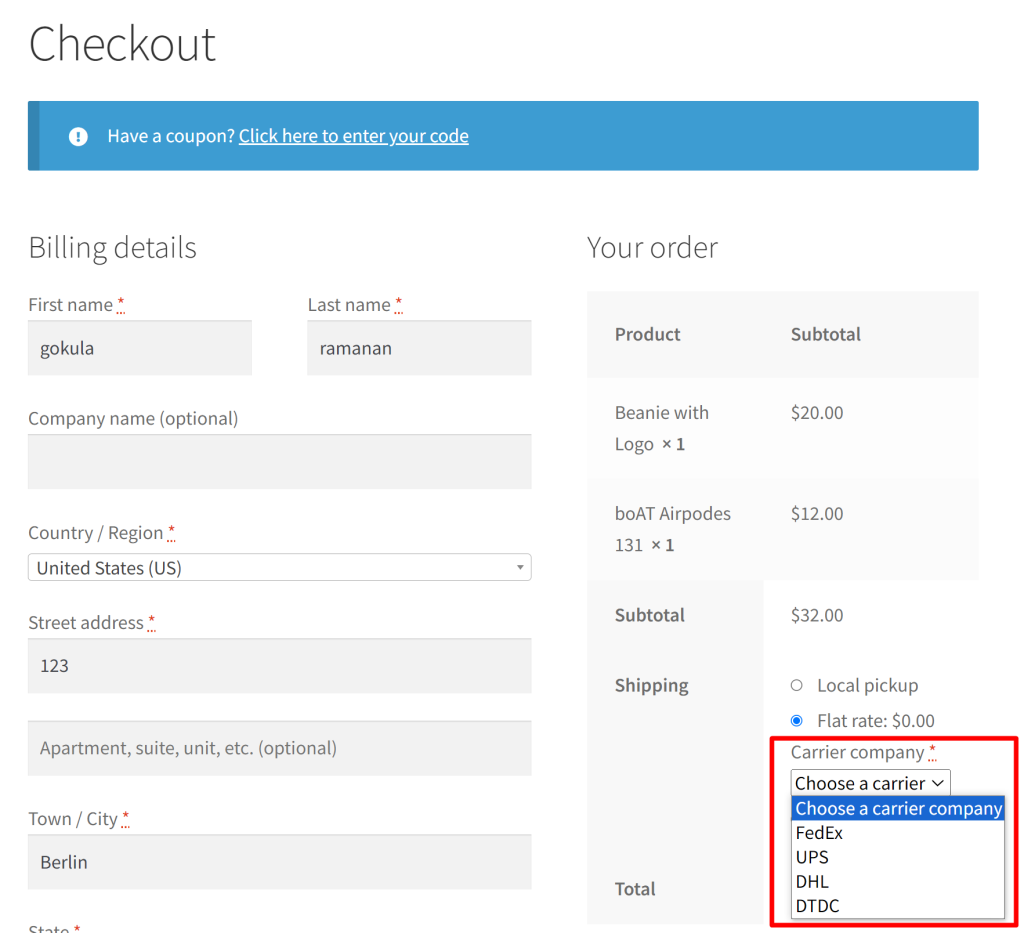
Use case: Shipping carrier selection and dynamic cost calculation
When the customer selects “FedEx” as their preferred carrier, an updated shipping cost of $15.00 is displayed on the shipping total and subsequently adjusts the total accordingly.
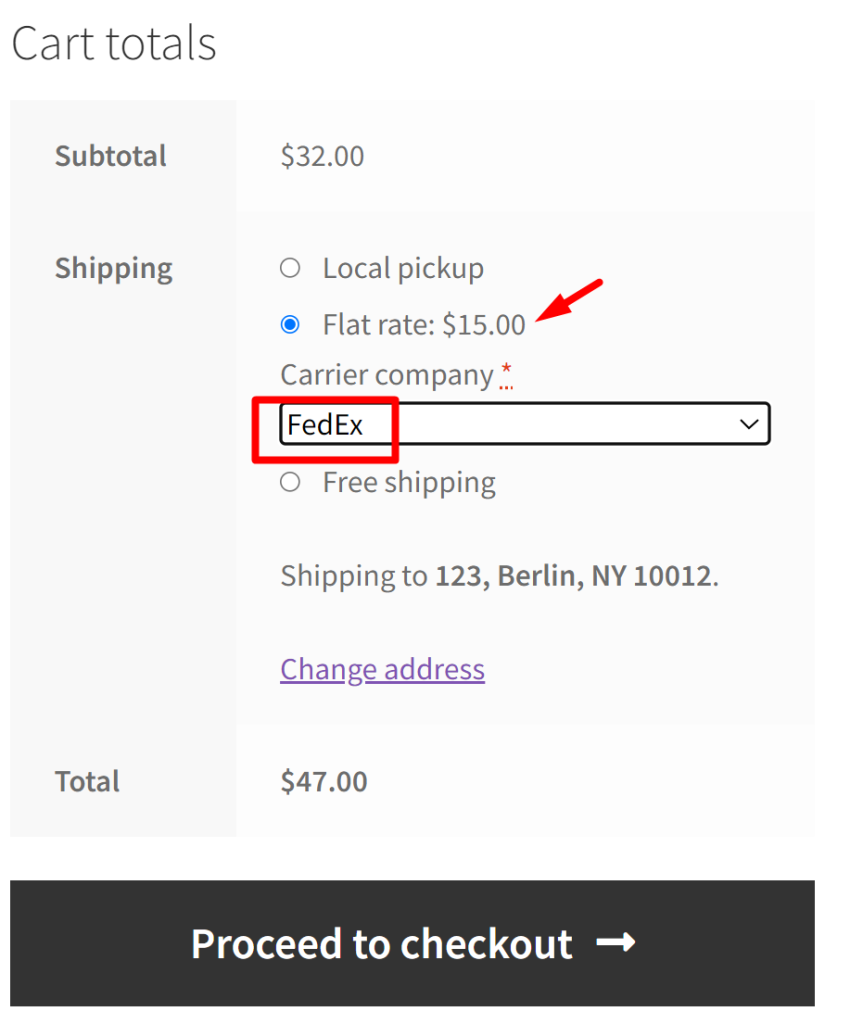
Code Explanation
1. ts_carrier_settings Function: This function defines the settings for your custom carrier field. It returns an array that includes:
- ‘targeted_methods’: An array specifying the targeted shipping method(s).
- ‘field_id’: The unique identifier for your carrier field.
- ‘field_type’: The type of field, in this case, it’s a select dropdown.
- ‘label_name’: The label for the carrier field, displayed for validation and as a meta key for orders.
- ‘field_options’: An array containing the carrier company names as keys and their respective flat rate values as values.
2. ts_carrier_company_custom_select_field Function: This function is hooked to woocommerce_after_shipping_rate. It handles the display of the custom carrier dropdown field. It does the following:
- Extracts the settings from the ts_carrier_settings function.
- Retrieves the chosen shipping methods and the selected carrier value from the session.
- Initializes an empty array called $options.
- Checks if the chosen shipping method matches the targeted method(s) and if the current method is in the $field_options array.
- If conditions are met, it displays the carrier dropdown field with options populated based on the carrier names in the $field_options array.
3. jQuery AJAX Handling (ts_carrier_company_script_js): This script is loaded in the footer and is used for handling AJAX requests when the carrier selection is changed. It performs the following actions:
- Check if the current page is the cart or checkout page.
- Extracts the settings from the ts_carrier_settings function.
- Determines the JavaScript variable ($js_variable) based on whether it’s the cart or checkout page.
- Listens for changes in the carrier dropdown field.
- When a change occurs, it sends an AJAX POST request to the server with the selected carrier value.
- Upon success, it parses the JSON response and updates the displayed shipping cost and cart total on the page.
4. WordPress AJAX Receiver (ts_update_shipping_rates): This function handles the server-side logic for updating shipping rates. It does the following:
- Extracts the settings from the ts_carrier_settings function.
- Retrieves the selected carrier value from the AJAX POST request.
- Looks up the flat rate value for the selected carrier from the $field_options array.
- Calculates the new cart total by adding the product subtotal and the new shipping rate.
- Sets the cart total to the new calculated total.
- Sends back a JSON response containing the new shipping rate and the updated cart total to the JavaScript for display.
Conclusion
The above code allows you to add a custom carrier selection field to your WooCommerce cart and checkout pages. When customers choose a carrier, it dynamically updates the displayed shipping cost and the cart’s total cost based on the selected carrier’s flat rate. You can modify the code to add a delivery date field for shipping methods in the WooCommerce cart and checkout page.