Based on the store’s specific business needs you can add custom fields to collect essential customer details from the registration form. Having additional details about customers enables store owners to send personalized promotions, discounts, or product recommendations based on the information collected during the registration process.
The code given below will add custom fields such as First name, Last name, Phone number, and country field to the WooCommerce registration page. Also, it will validate and save the field values that are entered and populate the values on the checkout page.
// Add extra fields to registration form add_action('woocommerce_register_form_start', 'ts_extra_register_fields'); function ts_extra_register_fields() { $countries_obj = new WC_Countries(); $countries = $countries_obj->get_countries(); // Pass `false` to get all countries // First Name woocommerce_form_field('billing_first_name', array( 'type' => 'text', 'class' => array('form-row-first'), 'label' => __('First name', 'woocommerce'), 'required' => true, )); // Last Name woocommerce_form_field('billing_last_name', array( 'type' => 'text', 'class' => array('form-row-last'), 'label' => __('Last name', 'woocommerce'), 'required' => true, )); // Phone Number woocommerce_form_field('billing_phone', array( 'type' => 'tel', 'class' => array('form-row-wide'), 'label' => __('Phone number', 'woocommerce'), 'required' => true, )); woocommerce_form_field('billing_country', array( 'type' => 'select', 'class' => array('chzn-drop'), 'label' => __('Country'), 'placeholder' => __('Choose your country'), 'options' => $countries, 'required' => true, )); } // Add JS code to My Account page footer add_action('wp_footer', 'ts_AccountPageJS', 100); function ts_AccountPageJS() { if (is_account_page()) { echo '<script>jQuery(".myClass").select2();</script>'; } } // Validate the additional fields add_filter('woocommerce_registration_errors', 'ts_validate_name_fields', 10, 3); function ts_validate_name_fields($errors, $username, $email) { if (isset($_POST['billing_first_name']) && empty($_POST['billing_first_name'])) { $errors->add('billing_first_name_error', __('<strong>Error</strong>: First name is required!', 'woocommerce')); } if (isset($_POST['billing_last_name']) && empty($_POST['billing_last_name'])) { $errors->add('billing_last_name_error', __('<strong>Error</strong>: Last name is required!', 'woocommerce')); } if (empty($_POST['billing_phone'])) { $errors->add('billing_phone_error', 'Phone is required!'); } return $errors; } // Save the additional fields add_action('woocommerce_created_customer', 'ts_save_name_fields'); function ts_save_name_fields($customer_id) { if (isset($_POST['billing_first_name'])) { update_user_meta($customer_id, 'billing_first_name', sanitize_text_field($_POST['billing_first_name'])); update_user_meta($customer_id, 'first_name', sanitize_text_field($_POST['billing_first_name'])); } if (isset($_POST['billing_last_name'])) { update_user_meta($customer_id, 'billing_last_name', sanitize_text_field($_POST['billing_last_name'])); update_user_meta($customer_id, 'last_name', sanitize_text_field($_POST['billing_last_name'])); } }
Output
The output shows that the registration form is filled with custom fields such as First name, last name, Phone number, and country field.
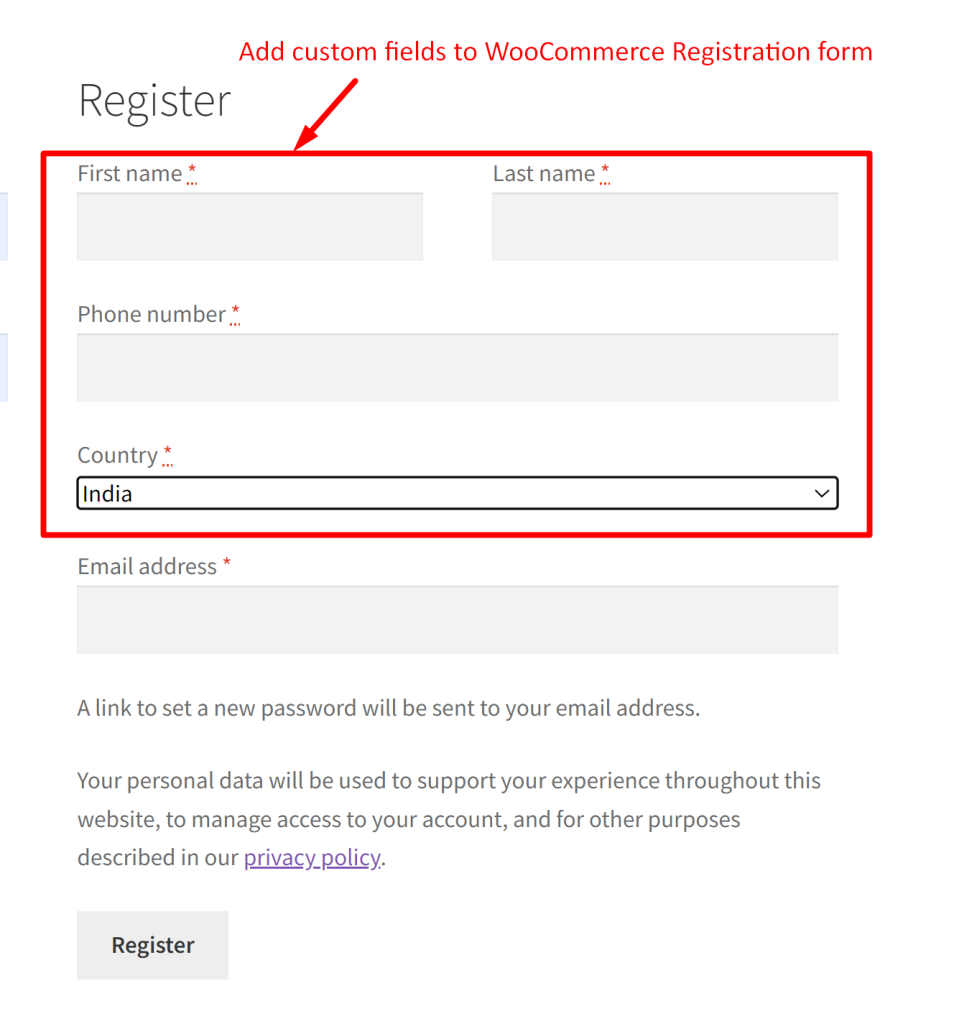
Let’s take a look at how the default registration form is presented on the WooCommerce ‘My Account ‘ page.
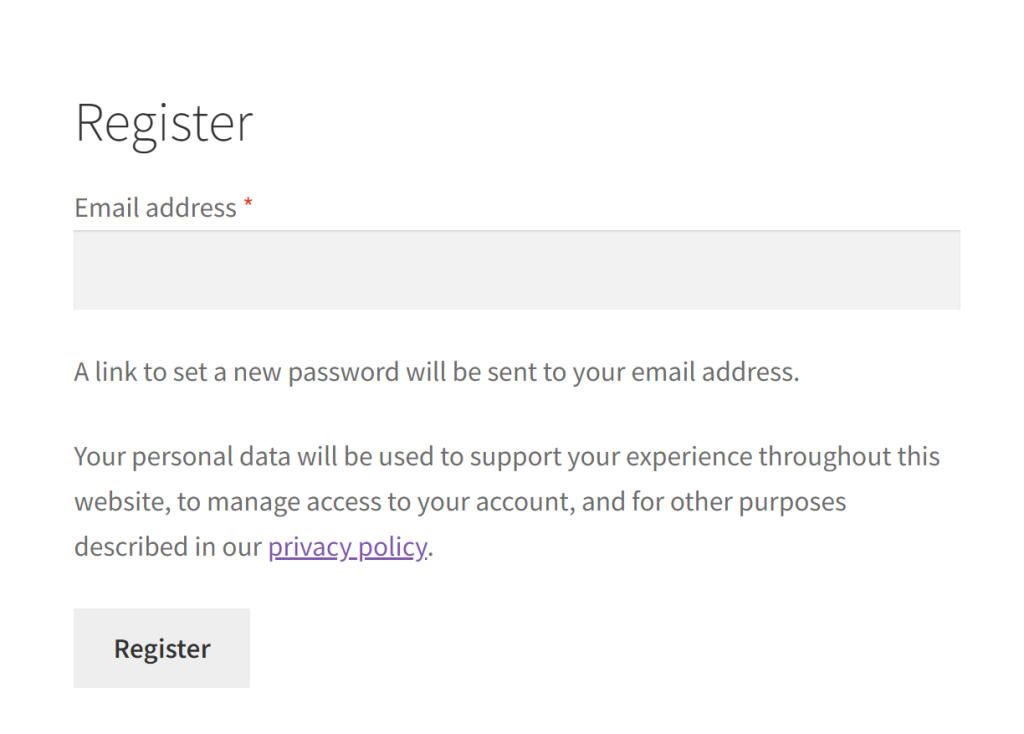
Additionally, you can also mask the phone number field on WooCommerce checkout which will display the country flag and country’s phone code as a dropdown to the phone field.
Similar to adding custom fields, you can also remove any fields if they don’t serve any purpose. Here is our guide that provides different solutions to remove last name field from my account page in WooCommerce.