As an online store owner, if you decide to restrict the quantity input field of certain products, you can take a step ahead and also restrict shipping for these products. For example when the quantity limit is set for the product with the IDs 100, 470, and 456 then when these products are present in the cart, the code tends to hide Free shipping for these products.
function ts_woocommerce_quantity_input_min_callback( $min, $product ) { // Array of product IDs for which to set the minimum quantity $product_ids = array( 100, 470, 457 ); // Update with your desired product IDs // Check if the current product ID is in the array if ( in_array( $product->get_id(), $product_ids ) ) { $min = 2; // Set the minimum quantity to 2 for the specified products } return $min; } add_filter( 'woocommerce_quantity_input_min', 'ts_woocommerce_quantity_input_min_callback', 10, 2 ); /* * Changing the maximum quantity to 5 for specific WooCommerce products */ function ts_woocommerce_quantity_input_max_callback( $max, $product ) { // Array of product IDs for which to set the maximum quantity $product_ids = array( 100, 470, 457 ); // Update with your desired product IDs // Check if the current product ID is in the array if ( in_array( $product->get_id(), $product_ids ) ) { $max = 5; // Set the maximum quantity to 5 for the specified products } return $max; } add_filter( 'woocommerce_quantity_input_max', 'ts_woocommerce_quantity_input_max_callback', 10, 2 ); // Hide free shipping when quantity input fields are restricted function ts_hide_free_shipping_with_quantity_restriction( $rates, $package ) { // Check if any of the restricted products are in the cart $restricted_product_ids = array( 100, 470, 457 ); // Same product IDs as in previous functions foreach ( $package['contents'] as $item ) { if ( in_array( $item['product_id'], $restricted_product_ids ) ) { // If restricted product found, remove free shipping rates foreach ( $rates as $rate_key => $rate ) { if ( 'free_shipping' === $rate->method_id ) { unset( $rates[ $rate_key ] ); } } break; // Stop looping as we found a restricted product } } return $rates; } add_filter( 'woocommerce_package_rates', 'ts_hide_free_shipping_with_quantity_restriction', 10, 2 );
Output
When a customer visits a product page of any of the restricted product IDs (e.g., 100
, 470
, 457
), the minimum and maximum quantity rules are applied to a minimum of 2
and a maximum of 5
. Subsequently, if he adds the products to the cart, then the code will tend to hide the specified shipping method ‘Free Shipping’.
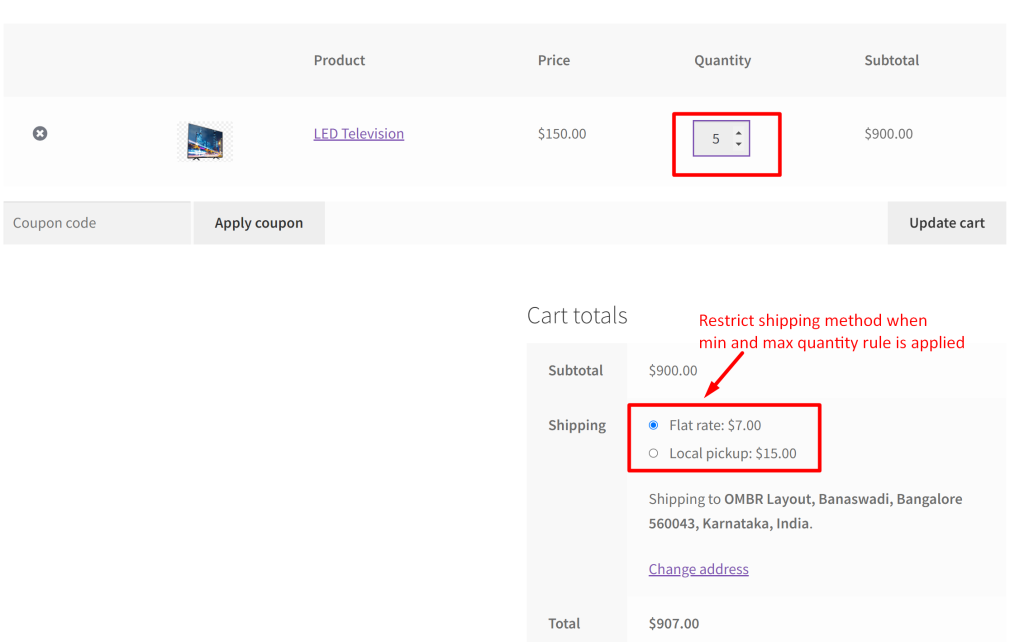
And if you wish to apply the min-max restriction rule to be applied even on the cart page then the below given code snippet must be added to the above code.
add_filter( 'woocommerce_package_rates', 'ts_hide_free_shipping_with_quantity_restriction', 10, 2 ); // Apply min and max quantity rules in cart page function ts_custom_cart_item_quantity( $product_quantity, $cart_item_key, $cart_item ) { $product_id = $cart_item['product_id']; $product_min = 2; // Set the minimum quantity $product_max = 5; // Set the maximum quantity // Check if the product is within the restricted product IDs $restricted_product_ids = array( 100, 470, 457 ); // Update with your desired product IDs if ( in_array( $product_id, $restricted_product_ids ) ) { // Apply minimum and maximum quantity rules $product_quantity = woocommerce_quantity_input( array( 'input_name' => "cart[{$cart_item_key}][qty]", 'input_value' => $cart_item['quantity'], 'max_value' => $product_max, 'min_value' => $product_min, 'product_name' => $cart_item['data']->get_name(), ), $cart_item['data'], false ); } return $product_quantity; } add_filter( 'woocommerce_cart_item_quantity', 'ts_custom_cart_item_quantity', 10, 3 );
Alternatively, you can also apply step increments as 2,4,6,8…etc and restrict the quantity field to selected numbers in WooCommerce.