Table of Contents
Are you planning to define the minimum and maximum quantities to the products which you are selling on your store build up with WooCommerce? If yes, then you can achieve your goal simply by using filters available in WooCommerce OR by using some plugins available on WordPress Repository.
First, we will see how to limit the minimum and maximum quantity of products that can be added to WooCommerce cart using the filters in WooCommerce. Below are the two filters which allow you to change the default min and max values for the quantity selector field.
- woocommerce_quantity_input_min : Allows to change the minimum value for the quantity. Default value is 0.
- woocommerce_quantity_input_max : Allows to change the maximum value for the quantity. Default value is -1.
/* * Changing the minimum quantity to 2 for all the WooCommerce products */ function woocommerce_quantity_input_min_callback( $min, $product ) { $min = 2; return $min; } add_filter( 'woocommerce_quantity_input_min', 'woocommerce_quantity_input_min_callback', 10, 2 ); /* * Changing the maximum quantity to 5 for all the WooCommerce products */ function woocommerce_quantity_input_max_callback( $max, $product ) { $max = 5; return $max; } add_filter( 'woocommerce_quantity_input_max', 'woocommerce_quantity_input_max_callback', 10, 2 );
So paste the above code snippet in your currently active theme’s functions.php file and visit the front end of the WooCommerce product page. You will be able to select quantity only between 2 to 5 for that item.
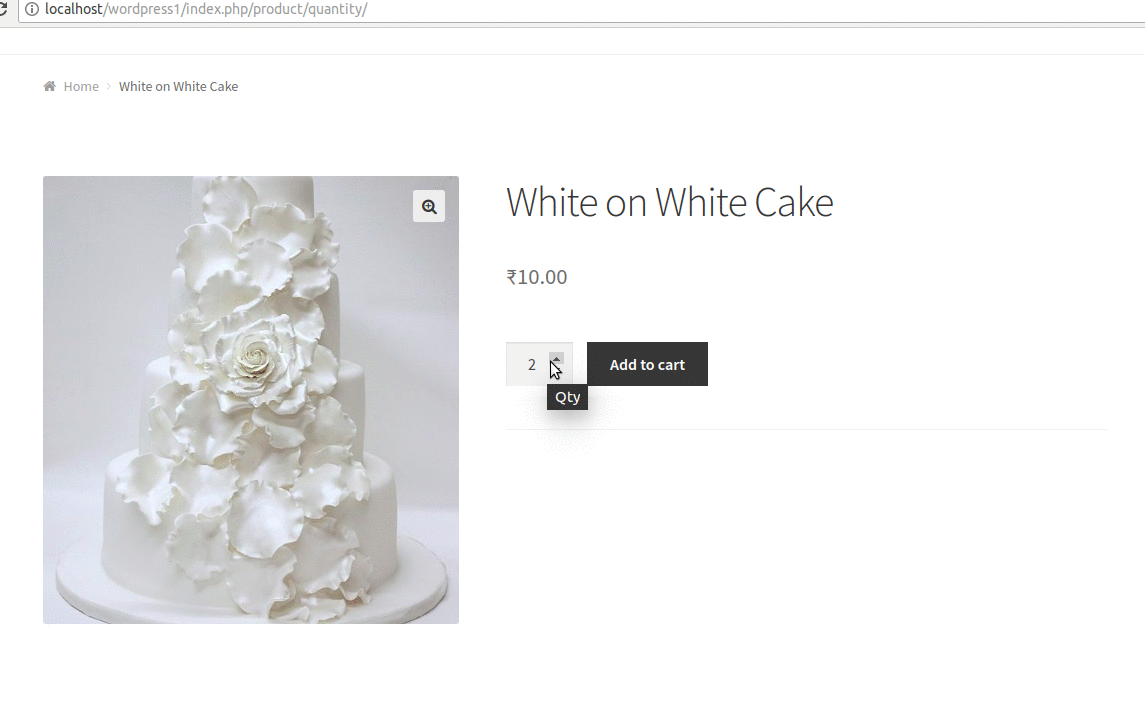
The above solution will only work on the WooCommerce product page. Which means, even after placing above code snippet in functions.php file, the customer can update the quantity on Cart page. Also, it will be applied to all the product available on your store.
To correct it, this will need some validations and some options where store owner can set the values to minimum and maximum quantity for the product.
So let’s create these options on the Product Meta box so that you can set this quantity for each product separately. And based on the values set to these options we can perform validations on the Cart page.
Where to Add Custom Code in WooCommerce?
In WordPress, adding the custom code can be done using the Theme Editor or via the Code Snippets plugin. Let’s see the steps on where to place the code.
1. Theme Editor:
- Navigate to Appearance > Theme Editor in your WordPress dashboard.
- Select the functions.php file from the list of theme files.
- Carefully insert the code snippet at the end of the file.
- Click the “Update File” button to apply the modifications.
2. Code Snippets Plugin:
- Go to Plugins > Add New and search for “Code Snippets.” Install a plugin called “Code Snippets” and activate it.
- Navigate to Snippets in your dashboard and click “Add New.”
- Paste the code snippet into the provided code editor.
- Give your snippet a descriptive title and click the “Save Changes and Activate” button.
Before implementing the code snippets, we need to find the ID of the particular variable product, so here are the steps as mentioned below.
- Navigate to Products > All Products.
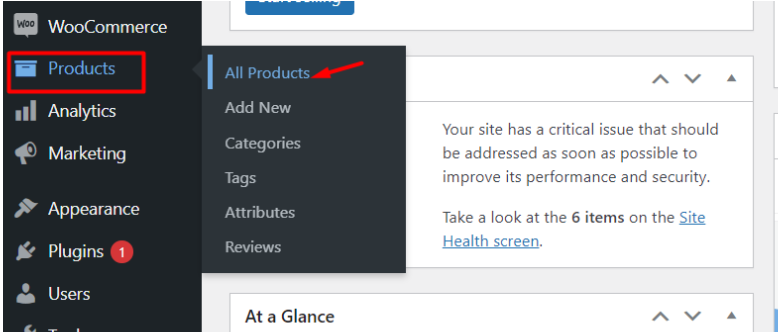
- After redirecting to the product page, click on the filter by product type dropdown select the option as the variable product, and click on the apply button.
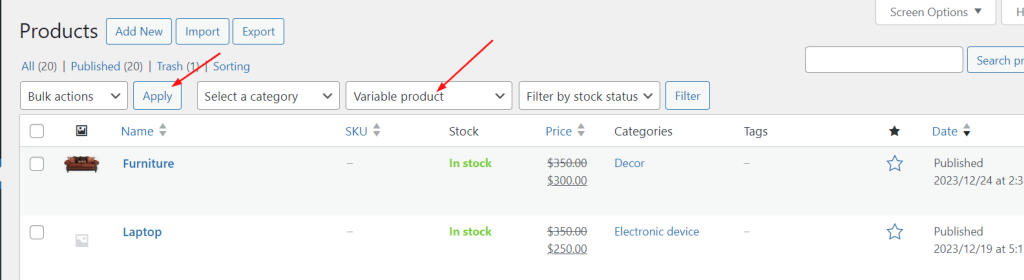
- Hover the cursor over the variable product name i.e. Hoodie and it will show the ID: 15 as shown below.
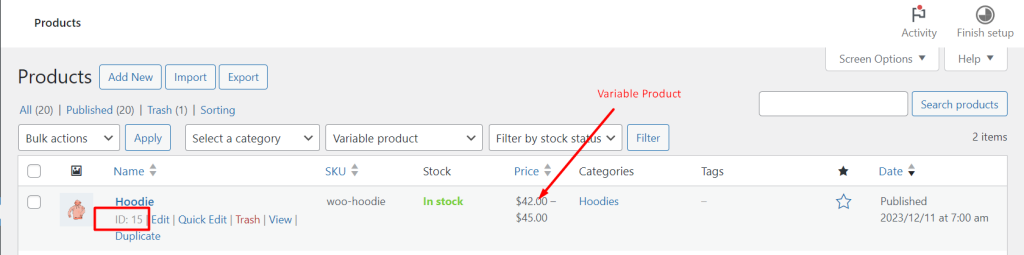
Set min and max quantities for the variable product
Imagine you run an online clothes store that sells clothes in various colors. You offer a variable product called “Hoodie” where customers can choose their favorite colors and also you want to set the minimum and maximum quantities for the variable product.
The below code snippets helps to modify the quantity field parameters for variable products.
add_filter( 'woocommerce_quantity_input_args', 'ts_min_max_qty_variable_products', 10, 2 ); function ts_min_max_qty_variable_products( $args, $product ) { $variation_product_id = array( 15 ); if ( ! is_cart() && in_array( $product->get_id(), $variation_product_id ) ) { $args['min_value'] = 3; $args['max_value'] = 6; } elseif ( is_cart() && in_array( $product->get_parent_id(), $variation_product_id ) ) { $args['min_value'] = 3; $args['max_value'] = 6; } return $args; }
Output
The output shows that the input field parameter is set to the minimum quantity of 3 and the maximum quantity of 6 for those specific variable product IDs specified in the code.
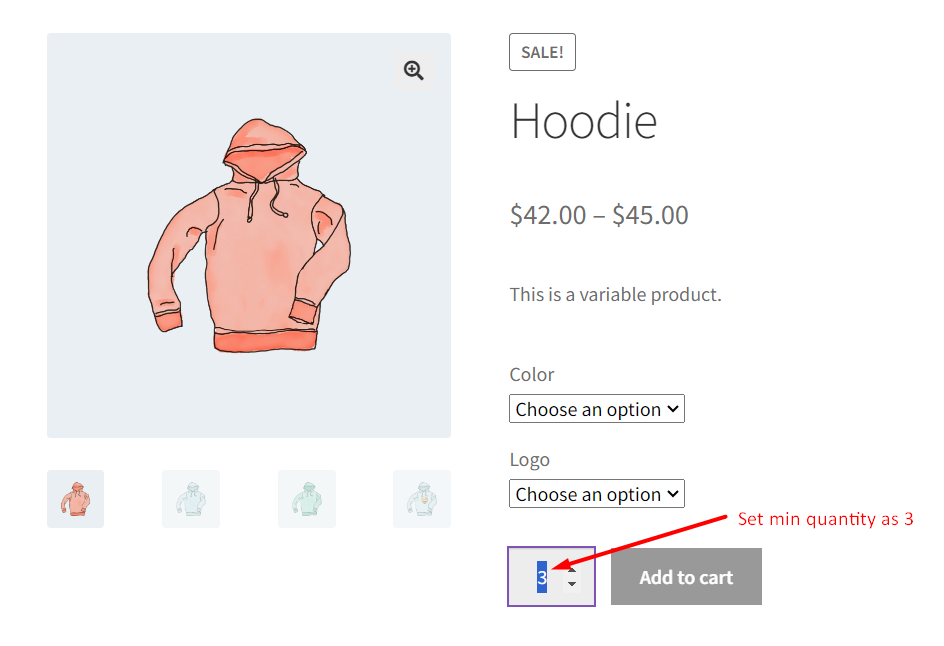
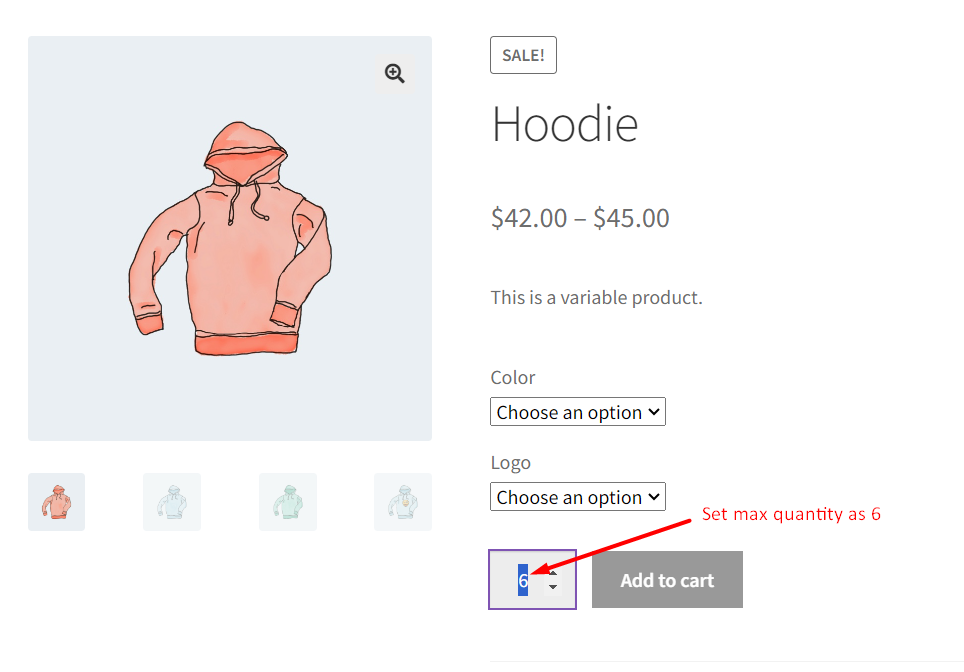
This code snippet connects to the quantity input arguments of WooCommerce and triggers a function called “ts_min_max_qty_variable_products” when the filter is applied. The function takes two parameters: $args and $product.
An array of variation product IDs is created with only one product with ID 15. If the current page is not the cart page and the product being considered has an ID that matches any in the $variation_product_id array, the minimum quantity is set to 3 and the maximum quantity to 6.
If the current page is the cart page and the parent product ID matches any in the $variation_product_id array, the minimum quantity is set to 3 and the maximum quantity to 6.
Finally, the function returns the modified quantity input arguments that will be used by WooCommerce to display the quantity input field based on the specified conditions.
Creation of Minimum & Maximum Quantity fields:
Inventory tab of Product data meta box will be the best place to add our options. Using woocommerce_product_options_inventory_product_data hook we can add extra fields at the end of options available in Inventory tab. Below is the code snippet which will add “Minimum Quantity” and “Maximum Quantity” options in the Inventory tab.
function wc_qty_add_product_field() { echo '<div class="options_group">'; woocommerce_wp_text_input( array( 'id' => '_wc_min_qty_product', 'label' => __( 'Minimum Quantity', 'woocommerce-max-quantity' ), 'placeholder' => '', 'desc_tip' => 'true', 'description' => __( 'Optional. Set a minimum quantity limit allowed per order. Enter a number, 1 or greater.', 'woocommerce-max-quantity' ) ) ); echo '</div>'; echo '<div class="options_group">'; woocommerce_wp_text_input( array( 'id' => '_wc_max_qty_product', 'label' => __( 'Maximum Quantity', 'woocommerce-max-quantity' ), 'placeholder' => '', 'desc_tip' => 'true', 'description' => __( 'Optional. Set a maximum quantity limit allowed per order. Enter a number, 1 or greater.', 'woocommerce-max-quantity' ) ) ); echo '</div>'; } add_action( 'woocommerce_product_options_inventory_product_data', 'wc_qty_add_product_field' );
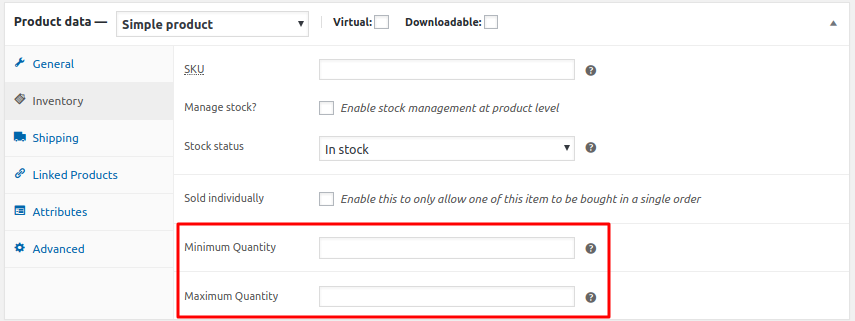
Next thing we need is saving the above options. Currently, they are only being shown on the Inventory section, but they won’t be saved if you enter values.
To save the values set to these options we will have to use woocommerce_process_product_meta action hook.
/* * This function will save the value set to Minimum Quantity and Maximum Quantity options * into _wc_min_qty_product and _wc_max_qty_product meta keys respectively */ function wc_qty_save_product_field( $post_id ) { $val_min = trim( get_post_meta( $post_id, '_wc_min_qty_product', true ) ); $new_min = sanitize_text_field( $_POST['_wc_min_qty_product'] ); $val_max = trim( get_post_meta( $post_id, '_wc_max_qty_product', true ) ); $new_max = sanitize_text_field( $_POST['_wc_max_qty_product'] ); if ( $val_min != $new_min ) { update_post_meta( $post_id, '_wc_min_qty_product', $new_min ); } if ( $val_max != $new_max ) { update_post_meta( $post_id, '_wc_max_qty_product', $new_max ); } } add_action( 'woocommerce_process_product_meta', 'wc_qty_save_product_field' );
When Publishing/Updating the product, the values set to “Minimum Quantity” and “Maximum Quantity” option will get saved in _wc_min_qty_product and _wc_max_qty_product meta keys of the post in the post_meta table.
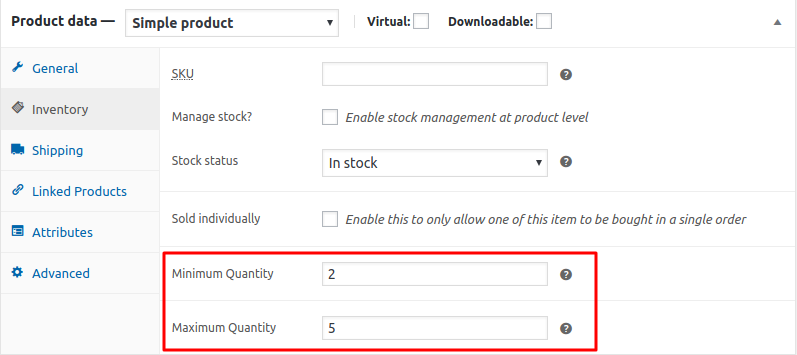
In the beginning of this post, we saw that we changed the minimum and maximum value for the quantity fields using the filter with some static values. In the below code we are fetching the values set to “Minimum Quantity” and “Maximum Quantity” and assigning it dynamically to arguments of quantity selector field.
/* * Setting minimum and maximum for quantity input args. */ function wc_qty_input_args( $args, $product ) { $product_id = $product->get_parent_id() ? $product->get_parent_id() : $product->get_id(); $product_min = wc_get_product_min_limit( $product_id ); $product_max = wc_get_product_max_limit( $product_id ); if ( ! empty( $product_min ) ) { // min is empty if ( false !== $product_min ) { $args['min_value'] = $product_min; } } if ( ! empty( $product_max ) ) { // max is empty if ( false !== $product_max ) { $args['max_value'] = $product_max; } } if ( $product->managing_stock() && ! $product->backorders_allowed() ) { $stock = $product->get_stock_quantity(); $args['max_value'] = min( $stock, $args['max_value'] ); } return $args; } add_filter( 'woocommerce_quantity_input_args', 'wc_qty_input_args', 10, 2 ); function wc_get_product_max_limit( $product_id ) { $qty = get_post_meta( $product_id, '_wc_max_qty_product', true ); if ( empty( $qty ) ) { $limit = false; } else { $limit = (int) $qty; } return $limit; } function wc_get_product_min_limit( $product_id ) { $qty = get_post_meta( $product_id, '_wc_min_qty_product', true ); if ( empty( $qty ) ) { $limit = false; } else { $limit = (int) $qty; } return $limit; }
Let’s understand what exactly is done in the above code snippet.
woocommerce_quantity_input_args is the filter which is used to alter the values being passed to create quantity field on the front end of the product page. Using this filter, in the callback wc_qty_input_args() function, we are setting the new values for min_value and max_value based on the values set in the “Minimum Quantity” and “Maximum Quantity” options.
You can now use the quantity limitations on the WooCommerce product page, which is only 1 part of the task.
Our second part is to have some validation on minimum and maximum quantity of the item when it is added to the cart and when it gets updated in the cart.
Validation on Add to Cart action
Let’s pause for a minute & think on why we need to validate on the add to cart action? For example, you have set the maximum quantity to 5 for Product A and you have added Product A for 5 quantity (max allowed quantity) in your basket. Now again you are adding Product A with 5 quantity and you are allowed to do this because there are no validations when the customer clicks on the “Add to Cart” button. That is why validation on the add to cart action is needed. Let’s see how we can do that.
WooCommerce has the woocommerce_add_to_cart_validation filter using which we can validate the add to cart action based on required conditions. As you can see in the below code snippet I have used the same filter. I
In the callback function wc_qty_add_to_cart_validation() I am checking that if the total quantity of the product in the cart is higher than the maximum quantity set for the product, then do not allow to add the product to the cart and display a WooCommerce error message.
/* * Validating the quantity on add to cart action with the quantity of the same product available in the cart. */ function wc_qty_add_to_cart_validation( $passed, $product_id, $quantity, $variation_id = '', $variations = '' ) { $product_min = wc_get_product_min_limit( $product_id ); $product_max = wc_get_product_max_limit( $product_id ); if ( ! empty( $product_min ) ) { // min is empty if ( false !== $product_min ) { $new_min = $product_min; } else { // neither max is set, so get out return $passed; } } if ( ! empty( $product_max ) ) { // min is empty if ( false !== $product_max ) { $new_max = $product_max; } else { // neither max is set, so get out return $passed; } } $already_in_cart = wc_qty_get_cart_qty( $product_id ); $product = wc_get_product( $product_id ); $product_title = $product->get_title(); if ( !is_null( $new_max ) && !empty( $already_in_cart ) ) { if ( ( $already_in_cart + $quantity ) > $new_max ) { // oops. too much. $passed = false; wc_add_notice( apply_filters( 'isa_wc_max_qty_error_message_already_had', sprintf( __( 'You can add a maximum of %1$s %2$s\'s to %3$s. You already have %4$s.', 'woocommerce-max-quantity' ), $new_max, $product_title, '<a href="' . esc_url( wc_get_cart_url() ) . '">' . __( 'your cart', 'woocommerce-max-quantity' ) . '</a>', $already_in_cart ), $new_max, $already_in_cart ), 'error' ); } } return $passed; } add_filter( 'woocommerce_add_to_cart_validation', 'wc_qty_add_to_cart_validation', 1, 5 ); /* * Get the total quantity of the product available in the cart. */ function wc_qty_get_cart_qty( $product_id ) { global $woocommerce; $running_qty = 0; // iniializing quantity to 0 // search the cart for the product in and calculate quantity. foreach($woocommerce->cart->get_cart() as $other_cart_item_keys => $values ) { if ( $product_id == $values['product_id'] ) { $running_qty += (int) $values['quantity']; } } return $running_qty; }
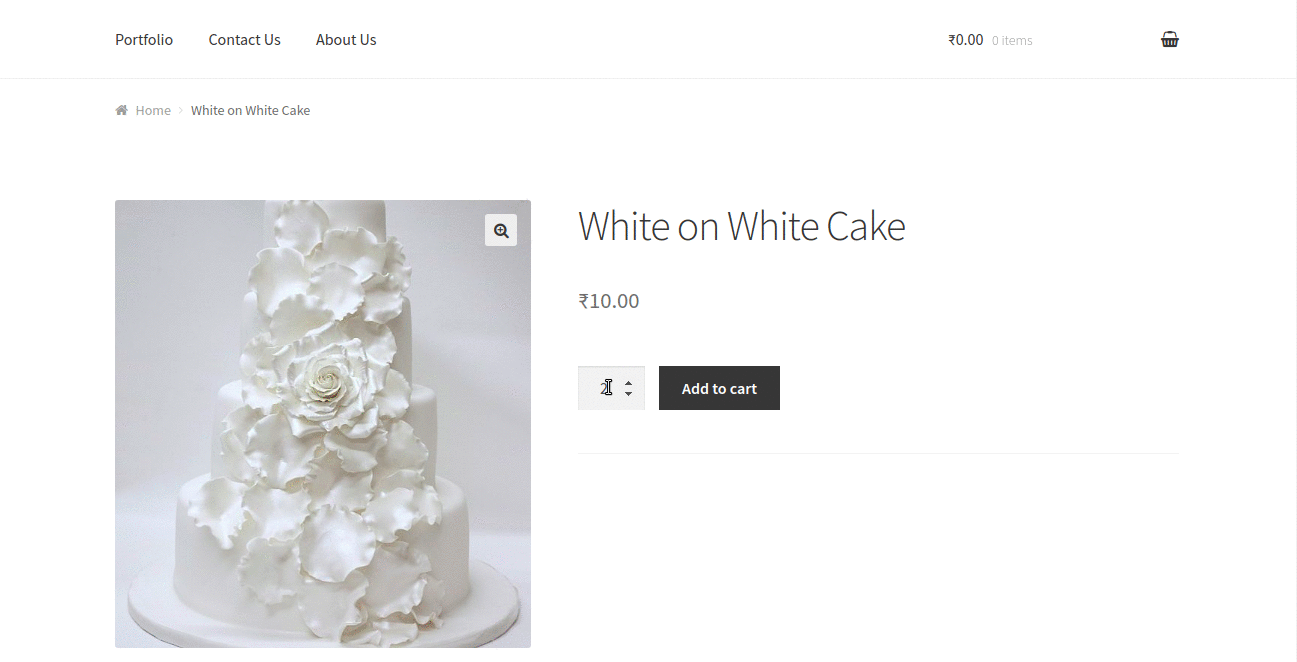
Validation on Updation of quantity in Cart page (woocommerce_update_cart_validation)
Again coming to the same question, why do we need to validate on Update Cart button action on the Cart page?
Because the customer can add Product A to the cart for 5 quantities and he is allowed to update the quantity higher than the value set to Minimum and Maximum Quantity options.
WooCommerce provides woocommerce_update_cart_validation filter to add the update cart action validations. Below is the code snippet which will do this validation.
/* * Get the total quantity of the product available in the cart. */ function wc_qty_get_cart_qty( $product_id , $cart_item_key = '' ) { global $woocommerce; $running_qty = 0; // iniializing quantity to 0 // search the cart for the product in and calculate quantity. foreach($woocommerce->cart->get_cart() as $other_cart_item_keys => $values ) { if ( $product_id == $values['product_id'] ) { if ( $cart_item_key == $other_cart_item_keys ) { continue; } $running_qty += (int) $values['quantity']; } } return $running_qty; } /* * Validate product quantity when cart is UPDATED */ function wc_qty_update_cart_validation( $passed, $cart_item_key, $values, $quantity ) { $product_min = wc_get_product_min_limit( $values['product_id'] ); $product_max = wc_get_product_max_limit( $values['product_id'] ); if ( ! empty( $product_min ) ) { // min is empty if ( false !== $product_min ) { $new_min = $product_min; } else { // neither max is set, so get out return $passed; } } if ( ! empty( $product_max ) ) { // min is empty if ( false !== $product_max ) { $new_max = $product_max; } else { // neither max is set, so get out return $passed; } } $product = wc_get_product( $values['product_id'] ); $already_in_cart = wc_qty_get_cart_qty( $values['product_id'], $cart_item_key ); if ( isset( $new_max) && ( $already_in_cart + $quantity ) > $new_max ) { wc_add_notice( apply_filters( 'wc_qty_error_message', sprintf( __( 'You can add a maximum of %1$s %2$s\'s to %3$s.', 'woocommerce-max-quantity' ), $new_max, $product->get_name(), '<a href="' . esc_url( wc_get_cart_url() ) . '">' . __( 'your cart', 'woocommerce-max-quantity' ) . '</a>'), $new_max ), 'error' ); $passed = false; } if ( isset( $new_min) && ( $already_in_cart + $quantity ) < $new_min ) { wc_add_notice( apply_filters( 'wc_qty_error_message', sprintf( __( 'You should have minimum of %1$s %2$s\'s to %3$s.', 'woocommerce-max-quantity' ), $new_min, $product->get_name(), '<a href="' . esc_url( wc_get_cart_url() ) . '">' . __( 'your cart', 'woocommerce-max-quantity' ) . '</a>'), $new_min ), 'error' ); $passed = false; } return $passed; } add_filter( 'woocommerce_update_cart_validation', 'wc_qty_update_cart_validation', 1, 4 );
As you can see in the above code snippet I have used the woocommerce_update_cart_validation filter to perform some validations based on the minimum and maximum quantity set for the product and the total quantity of that particular product available in the cart.
After this, the user cannot update the cart with a quantity less than the value set to the “Minimum Quantity” option and greater than the value set to the “Maximum Quantity” option.
In this post, we learn how to set minimum and maximum allowable product quantities to be added to WooCommerce Cart using custom code. You can add some additional options or conditions based on your business requirements.
Free & Premium WooCommerce plugins to set minimum & maximum quantity for products
There are paid plugins available that come with many other options to play with minimum and maximum allowed quantity and its rules. You can check the Minimum and Maximum Quantity for WooCommerce plugin which allows setting the Min/Max Quantity rule at the product level as well as at cart level. And it also allows setting minimum and maximum cart amount rules.
Below is the list of the free plugins available on WordPress.org which allows to set minimum and maximum allowable product quantities to be added in WooCommerce Cart.
1. Min and Max Quantity for WooCommerce
2. WooCommerce Minimum and Maximum Quantity
3. WooCommerce Max Quantity
Conclusion
Limiting the minimum & a maximum quantity of product can be achieved by using the filters provided by WooCommerce. Also, we have seen in this post that using some custom code snippets (including the filter available in WooCommerce) you can create proper interface and validations for allowing minimum and maximum quantity for the product. Or you can install already available plugins on your WordPress Repository and achieve the same.
In our Booking & Appointment plugin for WooCommerce, we added a feature recently where we are limiting the maximum allowed quantity for a product to be booked based on the Maximum booking set in our plugin. That’s where I got the idea to write this as a post.
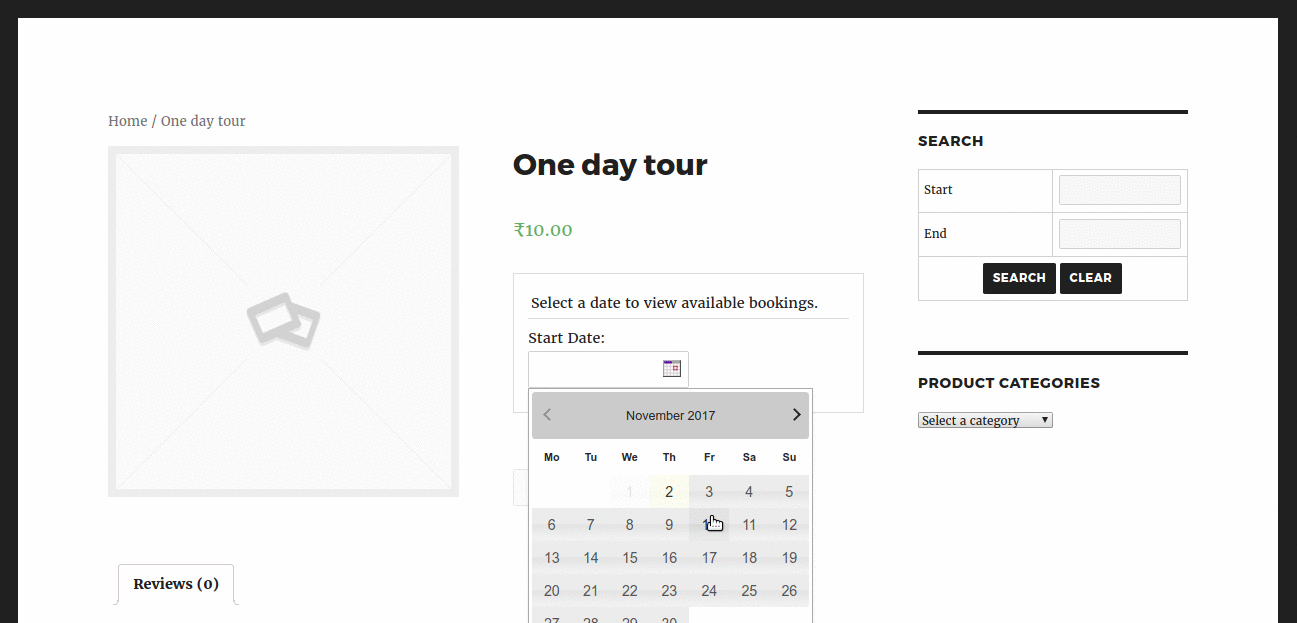
I hope this post helps you to learn how things in the WooCommerce can be easily customizable. If you have any questions then feel free to comment it.
This works perfectly. Most free plugins don’t allow you to set a limit per product, which is exactly what most. people need. Thanks a lot for the code and the insightful explanation of it.
My only suggestion would be to include all the code at the end. Now I had to copy and paste it from 4 fields. But this is minor.
Hello, why this code does not work for variable products?
Thank yyou
The max quantity is not applied on variabile products… does anyone encountered this problem?
Thank you.
Hi Vlad, The provided code snippet does not work for variable products. It only works for simple product types. If you’re looking to set the min/max for variable products. Here is the code snippet for setting the min/max for the product quantities. // Set min and max quantity for product variation add_filter( ‘woocommerce_quantity_input_args’, ‘wc_min_max_qty_variable_products’, 10, 2 ); function wc_min_max_qty_variable_products( $args, $product ) { $sample_product_id = array( 15 ); if ( ! is_cart() && in_array( $product->get_id(), $sample_product_id ) ) { $args[‘min_value’] = 3; $args[‘max_value’] = 6; } elseif ( is_cart() && in_array( $product->get_parent_id(), $sample_product_id ) ) { $args[‘min_value’] = 1; $args[‘step’]… Read more »
Hello can you please explain how to implement the function for variable products? thank you in advance
Hi Joe,
We have updated the post based on your request to implement the steps for setting the min/max quantity for variable products. Please refer to the heading “Where to Add Custom Code in WooCommerce?” and “Set min and max quantities for the variable product”
hi. I put all the codes that you put in the function file of my site. When I put the last piece of code, the site encountered a problem and was no longer available.
Validation on Updation of quantity in Cart page
Please guide me how to use these codes so that my site works properly
Hi mahsa,
I understand that your site went down after adding the code snippet for product quantity validation in the cart. Here’s how you can troubleshoot the issue:
By following these steps you can able to identify the cause of the problem of your site.
This is perfect, thanks a lot!
Thanks, this works perfectly!