In WooCommerce stores, the cart page isn’t just functional, but it also provides an opportunity to offer a more personalized shopping experience. On this page, customers are given a chance to review and modify their selected items or add the quantity of items before proceeding to checkout. Now, imagine if the cart page also suggests more related products based on what’s in the cart, customers may discover additional items and add them to their collections.
While the default options in the WooCommerce platform allow for some upselling and cross-selling, their capabilities are somewhat limited.
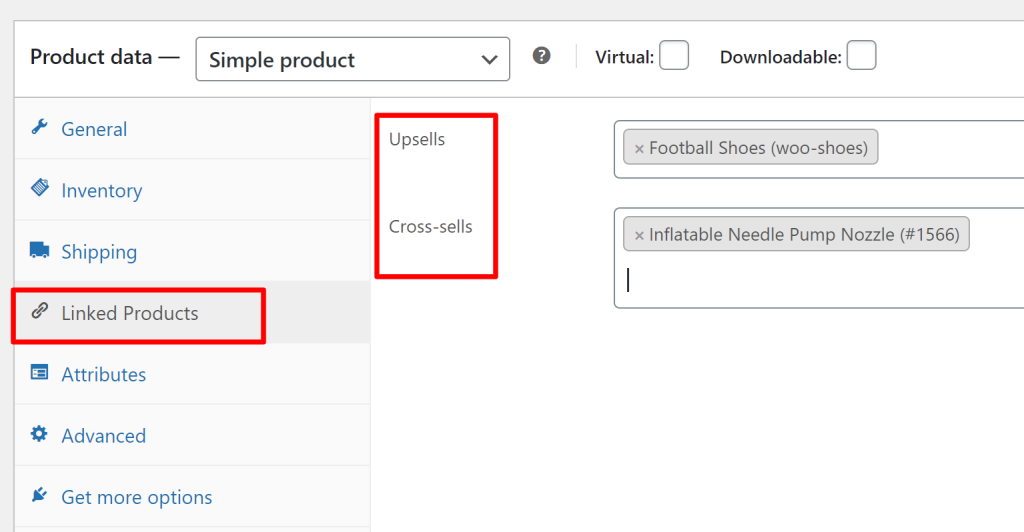
If you’re looking to establish a broader range of related product suggestions in your WooCommerce store, including upsells and cross-sells, you’ll find it necessary to utilize a plugin.
This post guides you on how to Show WooCommerce Related Products on the Cart Page using code snippets.
Where to Add Custom Code in WooCommerce?
It is advisable to add the code snippets to the functions.php file of your child theme. Access the file directly from Appearance->Theme File Editor->Locating the child theme’s functions.php from the right sidebar. You can also access it from your theme’s directory file. Insert the following code snippet in functions.php. The alternative & easy option is to install & activate the Code Snippets plugin. You can then add the code as a new snippet via the plugin.
Solution 1: Show Related Products for specific Category IDs on Cart Page in WooCommerce
Imagine an online store selling a wide range of items. The store owner wants to display a custom section on the cart page. This section will display related products and their links based on the specific product categories mentioned in the code snippet. Clicking on these related product links takes customers to the respective product pages. The code snippet provided below helps you to achieve this.
function ts_display_category_products_on_cart() { // Check if we are on the cart page if (is_cart()) { $category_ids = array(57, 58, 43); // Replace with your desired category IDs // Get the product categories for items in the cart $product_categories = array(); foreach (WC()->cart->get_cart() as $cart_item_key => $cart_item) { $product_id = $cart_item['product_id']; $terms = wp_get_post_terms($product_id, 'product_cat', array('fields' => 'ids')); $product_categories = array_merge($product_categories, $terms); } $product_categories = array_unique($product_categories); // Check if any product in the cart belongs to the specified categories $is_related_product = false; foreach ($category_ids as $term_id) { if (in_array($term_id, $product_categories)) { $is_related_product = true; break; } } if ($is_related_product) { foreach ($category_ids as $term_id) { // Check if any product in the cart belongs to the specified category if (in_array($term_id, $product_categories)) { $the_query = new WP_Query( array( 'post_type' => 'product', 'tax_query' => array( array( 'taxonomy' => 'product_cat', 'field' => 'id', 'terms' => $term_id, ), ), ) ); // Display the category title as a link $category = get_term($term_id, 'product_cat'); echo '<h2><a href="' . get_term_link($category) . '">Category: ' . $category->name . '</a></h2>'; while ( $the_query->have_posts() ) : $the_query->the_post(); // Display the product title as a link echo '<a href="' . get_permalink() . '">' . get_the_title() . '</a><br>'; endwhile; wp_reset_postdata(); } } } } } // Hook the custom function to the 'woocommerce_before_cart_table' action add_action( 'woocommerce_before_cart_table', 'ts_display_category_products_on_cart' );
Output
The output has listed the product links and the ‘Kitchen Appliance’s category link on the cart page. This is because we have explicitly mentioned this category ID also in the above code snippet. We would get such a custom section of related products only for the specified categories.
Code Explanation
- Function Definition:
- The code defines a custom PHP function named ts_display_category_products_on_cart. This function is responsible for displaying related products on the cart page.
- Check if on Cart Page:
- It checks whether the current page being viewed is the cart page using the is_cart() function. The code within this block executes only on the cart page.
- Define Category IDs:
- An array called $category_ids is defined, containing specific category IDs for which related products will be displayed. These IDs can be customized.
- Retrieve Product Categories in Cart:
- The code retrieves the product categories associated with items in the cart.
- It uses a foreach loop to iterate through each item in the cart.
- For each cart item, it extracts the product’s ID and retrieves its category terms.
- The category IDs are merged into the $product_categories array.
- Check for Related Products:
- It checks whether any product in the cart belongs to the specified categories.
- This is done by iterating through the $category_ids array and comparing them with the product categories in the cart.
- If a match is found, the $is_related_product flag is set to true.
- Display Related Products:
- If there are related products in the cart (based on the $is_related_product flag), the code enters a nested foreach loop to process each specified category.
- Create Custom Query for Related Products:
- Inside the loop, it checks if any product in the cart belongs to the current specified category.
- If so, it creates a custom query ($the_query) to retrieve products within that specific category.
- Display Category Title as a Link:
- For each category with related products in the cart, it displays the category title as a clickable link.
- This is achieved by obtaining category information using the get_term function and constructing an HTML link.
- Display Related Products Within the Category:
- Within the same category loop, the code iterates through the products obtained from the custom query ($the_query).
- For each product, it displays the product title as a clickable link, allowing users to navigate to individual product pages.
- Reset the Custom Query:
- After displaying related products for a specific category, the code resets the custom query ($the_query) using wp_reset_postdata(). This ensures subsequent operations are not affected.
- Hook the Function to the Cart Page:
The code concludes by hooking the ts_display_category_products_on_cart function to the woocommerce_before_cart_table action. This ensures that the function runs before the cart table is displayed on the cart page.
Solution 2: Show Related Products Based on Cart Items on Cart Page in WooCommerce
Instead of specific categories, we can also dynamically display product categories and related products on the cart page based on the products present in the cart. When a customer adds items to their cart, the code analyzes the products in the cart and extracts their respective categories and product links.
function ts_display_category_products_on_cart() { // Check if we are on the cart page if (is_cart()) { // Get the product categories for items in the cart $product_categories = array(); foreach (WC()->cart->get_cart() as $cart_item_key => $cart_item) { $product_id = $cart_item['product_id']; $terms = wp_get_post_terms($product_id, 'product_cat', array('fields' => 'ids')); $product_categories = array_merge($product_categories, $terms); } $product_categories = array_unique($product_categories); if (!empty($product_categories)) { foreach ($product_categories as $term_id) { $the_query = new WP_Query(array( 'post_type' => 'product', 'tax_query' => array( array( 'taxonomy' => 'product_cat', 'field' => 'id', 'terms' => $term_id, ), ), )); // Display the category title as a link $category = get_term($term_id, 'product_cat'); echo '<h2><a href="' . get_term_link($category) . '">Category: ' . $category->name . '</a></h2>'; while ($the_query->have_posts()) : $the_query->the_post(); // Display the product title as a link echo '<a href="' . get_permalink() . '">' . get_the_title() . '</a><br>'; endwhile; wp_reset_postdata(); } } } } // Hook the custom function to the 'woocommerce_before_cart_table' action add_action('woocommerce_before_cart_table', 'ts_display_category_products_on_cart');
Code Output
On the cart page, you can see a list of products and clickable links to their respective pages. These products belong to categories that match the items you’ve added to your cart. For example, if your cart contains items from the “Electronic Products” and “sports” category, you’ll find all related products from those categories listed on the cart page.
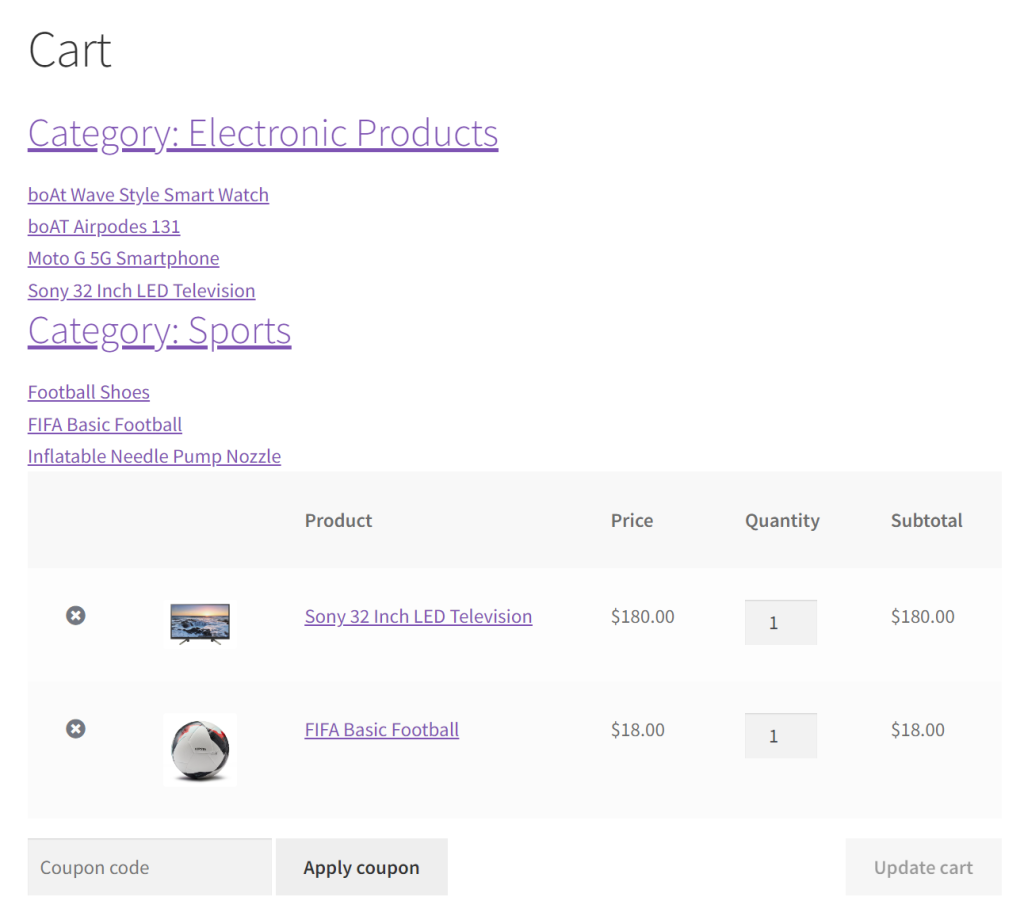
We are just doing some simple modifications in the above code to fetch related product categories of items present in the cart.
- Eliminating Hardcoded Category IDs: We removed the hardcoded $category_ids array, which previously contained specific category IDs. This change allows the code to dynamically determine the categories based on the products in the cart rather than relying on predefined IDs.
- Dynamic Category Retrieval: The code now dynamically fetches product categories from items in the cart. It iterates through the cart items, retrieves the associated categories, and stores them in the $product_categories array. This ensures that the code adapts to the actual products present in the cart.
- Conditional Category Display: By introducing the if (!empty($product_categories)) condition, the code ensures that it only proceeds to display categories and products if there are categories found in the cart. This avoids unnecessary output when the cart is empty or doesn’t contain products with categories.
Conclusion
By adjusting the hook and making a few code modifications, you can display products and their respective categories either on the shop page or the individual product pages. Alternatively, you can use custom code snippets to hide WooCommerce categories from being displayed on the shop page.
Let us know how the code was useful or any other queries in the comments section.