Table of Contents
Commonly, as a store owner, you may sometimes want to offer discounts if customers buy more than one quantity of a particular product. This may either be to boost sales or finish excess stocks. In this post, you will learn how to offer discounts based on quantity in WooCommerce using both code snippets and plugins.
How to offer discounts based on quantity in WooCommerce using Code Snippets
Let’s explore how to use a code snippet to do this. With this code snippet, we are offering customers a discount of 5% if they purchase over 2 quantities of the same product.
To do this, you will need to add the code below to the functions.php file of your child theme:
add_filter('woocommerce_add_cart_item_data', 'ts_add_default_price_as_custom', 20, 3 ); function ts_add_default_price_as_custom( $cart_item_data, $product_id, $variation_id ){ $product_id = $variation_id > 0 ? $variation_id : $product_id; ## ----- SET THE DISCOUNT HERE ----- ## $discount_percentage = 5; // Discount (5%) // The WC_Product Object $product = wc_get_product($product_id); $price = (float) $product->get_price(); // Set the Product default base price as custom cart item data $cart_item_data['base_price'] = $price; // Set the Product discounted price as custom cart item data $cart_item_data['new_price'] = $price * (100 - $discount_percentage) / 100; // Set the percentage as custom cart item data $cart_item_data['percentage'] = $discount_percentage; return $cart_item_data; } // Display the product original price add_filter('woocommerce_cart_item_price', 'ts_display_cart_items_default_price', 20, 3 ); function ts_display_cart_items_default_price( $product_price, $cart_item, $cart_item_key ){ if( isset($cart_item['base_price']) ) { $product = $cart_item['data']; $product_price = wc_price( wc_get_price_to_display( $product, array( 'price' => $cart_item['base_price'] ) ) ); } return $product_price; } // Display the product name with the discount percentage add_filter( 'woocommerce_cart_item_name', 'ts_add_percentage_to_item_name', 20, 3 ); function ts_add_percentage_to_item_name( $product_name, $cart_item, $cart_item_key ){ if( isset($cart_item['percentage']) && isset($cart_item['base_price']) ) { if( $cart_item['data']->get_price() != $cart_item['base_price'] ) $product_name .= ' <em>(' . $cart_item['percentage'] . '% discounted)</em>'; } return $product_name; } add_action( 'woocommerce_before_calculate_totals', 'ts_custom_discounted_cart_item_price', 20, 1 ); function ts_custom_discounted_cart_item_price( $cart ) { if ( is_admin() && ! defined( 'DOING_AJAX' ) ) return; if ( did_action( 'woocommerce_before_calculate_totals' ) >= 2 ) return; ## ----- SET THE QUANTITY HERE ----- ## $targeted_qty = 2; // Targeted quantity // Loop through cart items foreach ( $cart->get_cart() as $cart_item ) { // For item quantity of 2 or more if( $cart_item['quantity'] >= $targeted_qty && isset($cart_item['new_price']) ){ // Set cart item discounted price $cart_item['data']->set_price($cart_item['new_price']); } } }
The above code will add a discount of 5% if the quantity of the product is above 2. You can change this to suit your requirements by changing the $discount_percentage variable to set the desired discount, and the $targeted_qty to set the quantity above which you wish to apply the discount.
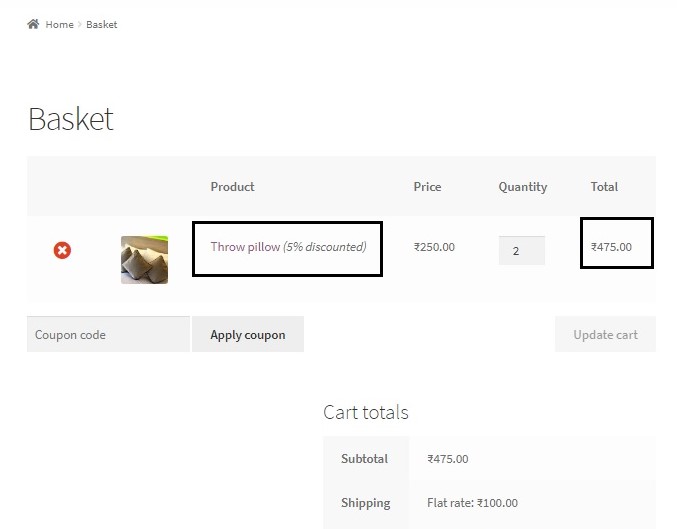
You will now be able to see a discounted price if you add 2 or more quantities of the same product in your cart.
We have used four major hooks in the above code:
- woocommerce_add_cart_item_data : This filter hook is used to change the contents / meta data of the cart.
- woocommerce_cart_item_price : This filter hook is used to change the way prices are displayed in the cart.
- woocommerce_cart_item_name : This filter hook can be used to change the product name in the cart. In our example, we are using it to append the discount percentage to the product name.
- woocommerce_before_calculate_totals : This action hook is used to override the product price in the cart.
How to offer discounts based on quantity only for items that are NOT on Sale:
If you want to restrict this to only those products which are not on sale, you simple have to edit the code snippet above to add a condition inside the function that is added to the hook woocommerce_add_cart_item_data:
add_filter('woocommerce_add_cart_item_data', 'ts_add_default_price_as_custom', 20, 3 ); function ts_add_default_price_as_custom( $cart_item_data, $product_id, $variation_id ){ $product_id = $variation_id > 0 ? $variation_id : $product_id; ## ----- SET THE DISCOUNT HERE ----- ## $discount_percentage = 5; // Discount (5%) // The WC_Product Object $product = wc_get_product($product_id); // ADD CONDITION HERE to offer DISCOUNT ONLY for NON On-Sale Products if( ! $product->is_on_sale() ){ $price = (float) $product->get_price(); // Set the Product default base price as custom cart item data $cart_item_data['base_price'] = $price; // Set the Product discounted price as custom cart item data $cart_item_data['new_price'] = $price * (100 - $discount_percentage) / 100; // Set the percentage as custom cart item data $cart_item_data['percentage'] = $discount_percentage; } return $cart_item_data; } // Display the product original price add_filter('woocommerce_cart_item_price', 'ts_display_cart_items_default_price', 20, 3 ); function ts_display_cart_items_default_price( $product_price, $cart_item, $cart_item_key ){ if( isset($cart_item['base_price']) ) { $product = $cart_item['data']; $product_price = wc_price( wc_get_price_to_display( $product, array( 'price' => $cart_item['base_price'] ) ) ); } return $product_price; } // Display the product name with the discount percentage add_filter( 'woocommerce_cart_item_name', 'ts_add_percentage_to_item_name', 20, 3 ); function ts_add_percentage_to_item_name( $product_name, $cart_item, $cart_item_key ){ if( isset($cart_item['percentage']) && isset($cart_item['base_price']) ) { if( $cart_item['data']->get_price() != $cart_item['base_price'] ) $product_name .= ' <em>(' . $cart_item['percentage'] . '% discounted)</em>'; } return $product_name; } add_action( 'woocommerce_before_calculate_totals', 'ts_custom_discounted_cart_item_price', 20, 1 ); function ts_custom_discounted_cart_item_price( $cart ) { if ( is_admin() && ! defined( 'DOING_AJAX' ) ) return; if ( did_action( 'woocommerce_before_calculate_totals' ) >= 2 ) return; ## ----- SET THE QUANTITY HERE ----- ## $targeted_qty = 2; // Targeted quantity // Loop through cart items foreach ( $cart->get_cart() as $cart_item ) { // For item quantity of 2 or more if( $cart_item['quantity'] >= $targeted_qty && isset($cart_item['new_price']) ){ // Set cart item discounted price $cart_item['data']->set_price($cart_item['new_price']); } } }
In this way, you can offer discounts based on quantity using code snippets for non-sale, sale or all products i.e. store-wide by stating the appropriate condition inside the function that is added to the filter hook woocommerce_add_cart_item_data.
How to offer discounts based on quantity in WooCommerce using Plugins
If you are not comfortable with adding a code snippet to your website files, you can use plugins to do this too:
1. YayPricing – WooCommerce Dynamic Pricing & Discounts
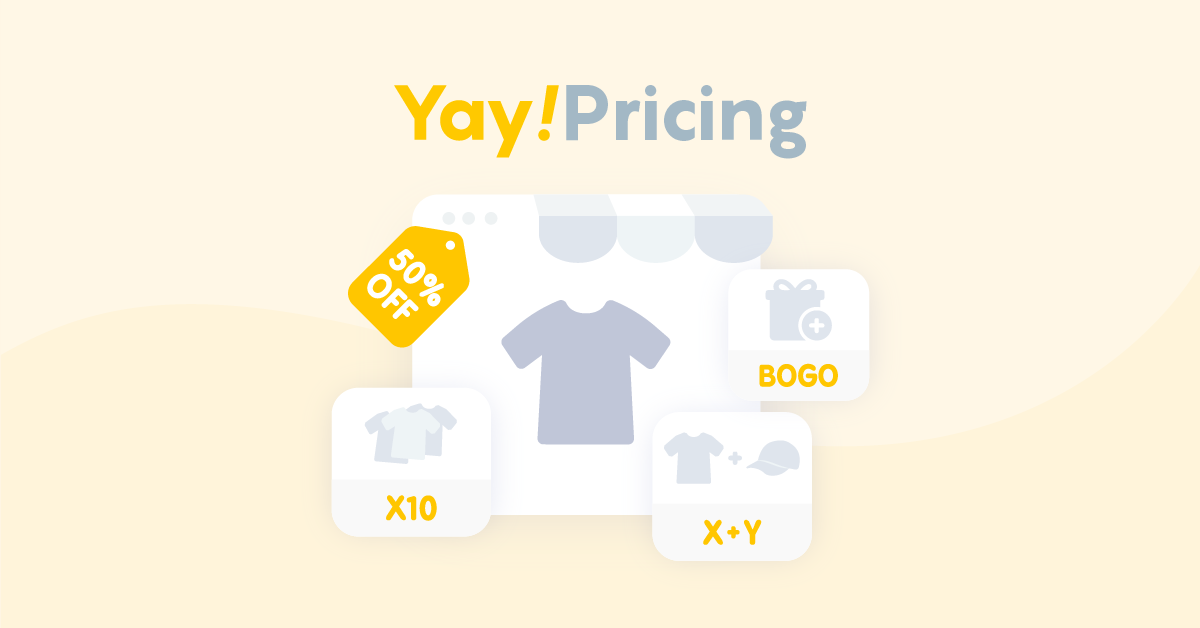
YayPricing allows you to set up scheduled discounts, bulk pricing, BOGO – buy one get one free, buy X get Y, discounts based on cart conditions, and much more.
The extension comes with a flexible pricing engine that makes it easy to create complex pricing rules without coding. WooCommerce Dynamic Pricing & Discounts by YayPricing is an efficient way to boost your store’s sales and revenues.
- Insert messages and sale banners to the product page (above add to cart button).
- Drag and drop interface with compact and collapsible cards.
- Countdown timer, free shipping notice, tiered discount table, etc
2. Discount Rules for WooCommerce
This is a free plugin that lets you add percentage discounts not just based on quantities of products, but also if your customers exceed a certain price limit. You can also add percentage discounts on a certain number of items (not necessarily of the same product). The PRO version of this plugin allows you to add category-wide discounts, BOGO (Buy One Get One Free) deals, a fixed price discount instead of a percentage discount, and more.
3. Conditional Discounts for WooCommerce
This is another plugin that offers nearly all important features in its free version- You can add discounts based on quantity, number of cart items, for specific categories, on specific products, on variable features (blue shirt, size Medium etc.), for certain shop roles or even specific customers. Its PRO version has a few additional features one of them being adding a product free of cost to the cart on purchase of a certain product.
4. Smart Coupons
Apart from gift cards, store credits, URL coupons and advanced restrictions, you can also restrict a coupon based on the number of specific products in the cart (example – 10 shirts) and also the total product quantity of multiple products in the cart (example – 5 shirts + 2 pants + 3 belts).
In this way, you may use either code snippets or plugins to offer discounts to your customers based on quantities of products.
Above code snippet for discounts is buggy. Discounts not reliably shown on the basket/cart page. For example, if the targeted amount is 5 and and the cart is increased to 5, the discount is not shown unless the page is refreshed. However, it will be shown when increased to 6 without refreshing the page (!?) Similarly, the discount will still be shown if the cart is then reduced to 4 unless the page is refreshed, but is no longer shown when reduced to 3. Thus it seems that 2+or- from the target amount is registered, but not 1+or-. Is this… Read more »
Hi Mark,
I’ve tested the code and the ‘Discount Rules for WooCommerce‘ plugin in the updated version of WooCommerce 8.5.1. And both seem to display the discount amount correctly when you click the “Update Cart” button. If you’re facing caching issues, it might be due to theme or plugin conflicts. Try disabling them temporarily and ensure your WordPress/WooCommerce installations are up to date.
Hi, Thanks for the reply. Using the ‘Discount Rules for Woocommerce’ plugin and changing settings, I found that the cart page would show the discount strikeout and wrong cart total when reduced by 1 from the target amount. This behaviour persisted even when theme was changed and all other plugins (apart from woocommerce ones) were deactivated. I did find that changing a setting on the discount rule solved the problem for me. This was the ‘Show discount in cart as coupon instead of changing the product price?’ setting. When this was ticked, the discount no longer showed when reducing the… Read more »
Hi Mark,
I tried to reproduce the same steps in the plugin settings as you mentioned in the comment and the discount price and the cart total is getting updated correctly. Please refer to the settings to refer to the options that I have enabled in the screenshot (https://prnt.sc/-cy24f53aDlh) and the frontend output (https://prnt.sc/OmL4ntApr3n8). On increasing/decreasing the quantity field the ‘update button’ is also shown. As this is a plugin-specific issue, I would recommend you contact the plugin author.
Modify to do amount discount? I’ll play around with it some, but basically want to discount of 50¢ each for 10 or more Widgets purchased.
Widget cost $1 each.
If 9 bought = $9
if 10 = $8.50; if 11 = $10;
If 20 widgets purchased, = $14.50
Can you discount an amount not a percentage? I’ll play around with it to check, but want to do a discount of 50¢ each for 10 or more Widgets purchased.
Widget cost $1 each.
If 9 bought = $9
if 10 = $8.50; if 11 = $10;
If 20 widgets purchased, = $14.50
Thanks for this., Any idea how to modify the code so it works when there’s between 1 – 5 products
Love this snippet! What would I need to change about it to restrict it to only those products I specify? Thanks!