Running an online store often involves regularly providing coupons and discounts for special events. While these discounts are a treat for customers, why not make the process even more convenient for them? Applying coupons directly through a code eliminates the requirement for customers to remember or manually input coupon codes during the WooCommerce checkout process.
This post will guide you on how to apply coupons programmatically in WooCommerce.
Where to Add Custom Code in WooCommerce?
It is advisable to add the code snippets to the functions.php file of your child theme. Access the file directly from Appearance->Theme File Editor->Locating the child theme’s functions.php from the right sidebar. You can also access it from your theme’s directory file. Insert the following code snippet in functions.php. The alternative & easy option is to install & activate the Code Snippets plugin. You can then add the code as a new snippet via the plugin.
Solution 1: Apply coupon for orders over a certain amount
Suppose you’re running an online store that sells various products and you want to encourage customers to make larger purchases by offering free shipping for orders over a certain amount, say $50. You can use the provided snippets to automatically apply a coupon code such as ‘freeshipping50’ to carts that meet the specified minimum order amount.
add_action( 'woocommerce_before_cart', 'ts_apply_coupon' ); function ts_apply_coupon() { $coupon_code = 'freeshipping50'; $cart_total = WC()->cart->get_total(); if ($cart_total >= 50) { if ( WC()->cart->has_discount( $coupon_code ) ) return; WC()->cart->apply_coupon( $coupon_code ); wc_print_notices(); } }
Output
If a customer adds products to their cart and the cart total reaches a certain amount, the coupon code will be applied automatically.
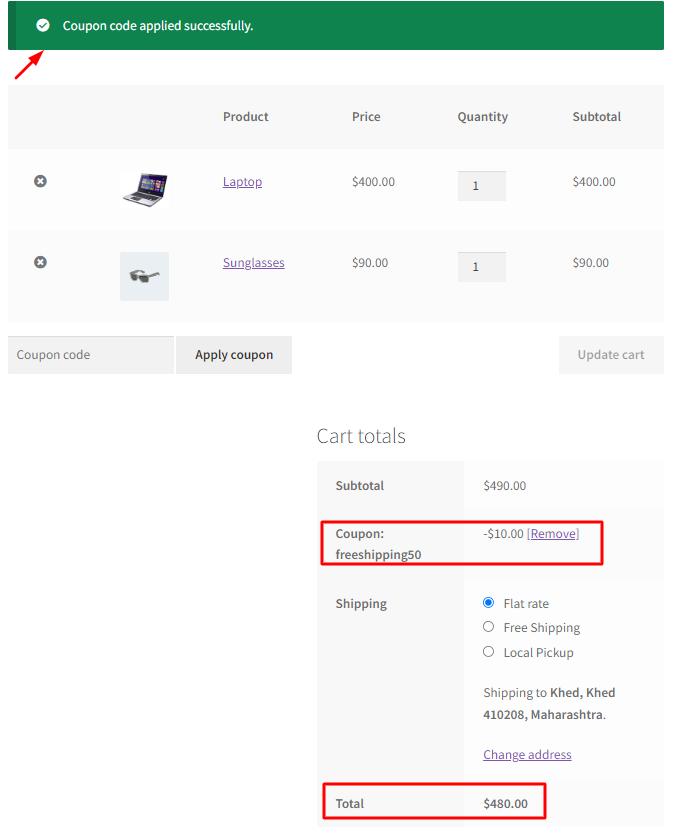
Code Explanation
- add_action( ‘woocommerce_before_cart’, ‘ts_apply_coupon’ );: This line adds an action hook to WordPress. Specifically, it hooks into the woocommerce_before_cart action, which is triggered before the WooCommerce shopping cart is displayed. When this action is triggered, it will call the ts_apply_coupon function.
- function ts_apply_coupon() { … }: This is the definition of the ts_apply_coupon function, which contains the logic for applying a coupon code to the WooCommerce cart.
- $coupon_code = ‘freeshipping50’;: This line defines the coupon code that the function will attempt to apply to the cart. In this case, the coupon code is set to ‘freeshipping50‘.
- $cart_total = WC()->cart->get_total();: This line retrieves the total value of the items in the WooCommerce cart. The WC()->cart object is used to access the cart-related functions and properties, and get_total() gets the total cart value.
- if ($cart_total >= 50) { … }: This is a conditional statement that checks if the cart total is greater than or equal to $50. If the condition is met, the code within this block will be executed.
- if ( WC()->cart->has_discount( $coupon_code ) ) return; This line checks whether the cart already has the specified coupon code applied. If the coupon code is already applied, the function exits early (using return) without applying it again to avoid duplicating the discount.
- WC()->cart->apply_coupon( $coupon_code );: If the cart total is $50 or more, and the coupon code is not already applied, this line applies the coupon code ‘freeshipping50’ to the cart. This provides a free shipping discount when the cart total meets the condition.
- wc_print_notices();: After applying the coupon code, this function is called to print any notices or messages related to the coupon code application. These notices may include messages like “Coupon applied successfully” or any other relevant information for the user.
Solution 2: Apply a coupon when a specific product is added to cart
Consider you want to run a promotion where customers receive a 20% discount on a particular product, say a new smartphone model. You can use the provided snippets to apply the coupon automatically when the specific product is added to the cart.
add_action( 'woocommerce_before_cart', 'ts_apply_coupon' ); function ts_apply_coupon() { $coupon_code = 'smartphonediscount20'; $product_id = 487; // Replace with the actual product ID if ( WC()->cart->has_discount( $coupon_code ) ) return; foreach ( WC()->cart->get_cart() as $cart_item_key => $cart_item ) { if ($cart_item['product_id'] == $product_id) { WC()->cart->apply_coupon( $coupon_code ); wc_print_notices(); break; } } }
Output
If a customer adds a specific product, namely iPhone 12 (product ID: 487), to their cart, the coupon code will be applied automatically.
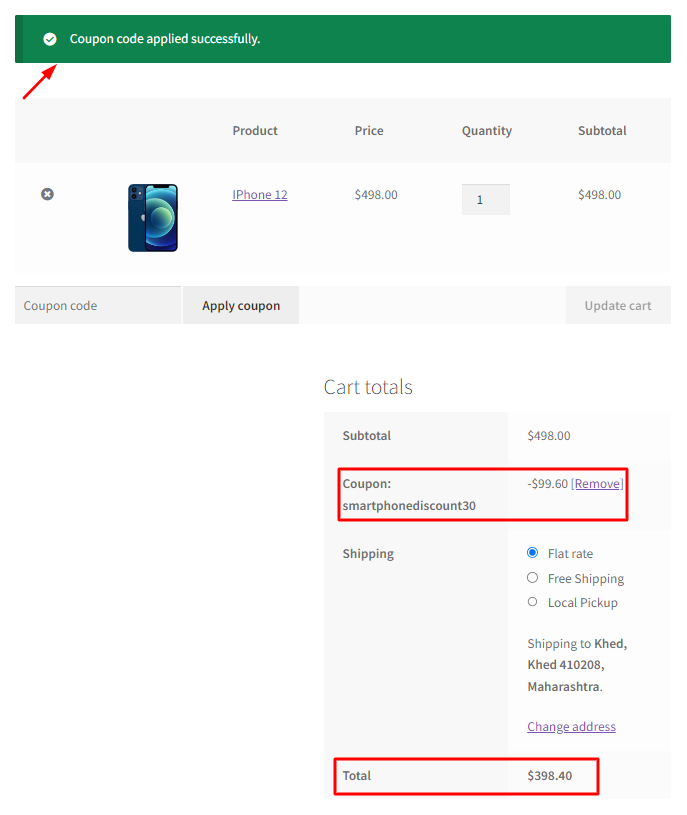
Code Explanation
- The code uses the add_action function to hook into the woocommerce_before_cart action. When the WooCommerce cart is about to be displayed, it calls the ts_apply_coupon function.
- The function defines a coupon code ($coupon_code) and a specific product ID ($product_id) that you want to check for in the cart.
- It checks whether the specified coupon code is already applied to the cart to avoid duplicate discounts.
- The code iterates through the items in the WooCommerce cart using a foreach loop, checking each item’s product ID.
- If the specific product is found in the cart, it applies the ‘smartphonediscount20’ coupon code and displays relevant notices using wc_print_notices.
Solution 3: Apply coupon depending on the number of products in the cart
Imagine you’re running an online store selling trendy fashion items. You want to encourage customers to purchase more products, not just focus on single items. You want to offer a special discount of “blackfrd” for carts containing more than 3 products. Below is the code snippet for this scenario.
// Hook the 'ts_apply_coupon_if_number_of_products' function to the 'woocommerce_before_cart' action add_action( 'woocommerce_before_cart', 'ts_apply_coupon_if_number_of_products' ); // Define the 'ts_apply_coupon_if_number_of_products' function function ts_apply_coupon_if_number_of_products() { // Set the coupon code to 'blackfrd' $coupon_code = 'blackfrd'; // Check if there are more than 3 different products in the cart if ( count( WC()->cart->get_cart() ) > 3 ) { // If the coupon is not already applied, apply it if ( ! WC()->cart->has_discount( $coupon_code ) ) { WC()->cart->apply_coupon( $coupon_code ); } } else { // If there are 3 or fewer different products, remove the coupon if ( WC()->cart->has_discount( $coupon_code ) ) { WC()->cart->remove_coupon( $coupon_code ); } } // Recalculate the cart totals WC()->cart->calculate_totals(); }
Output
When the customer adds more than 3 products to the cart, then the coupon code will get automatically applied.
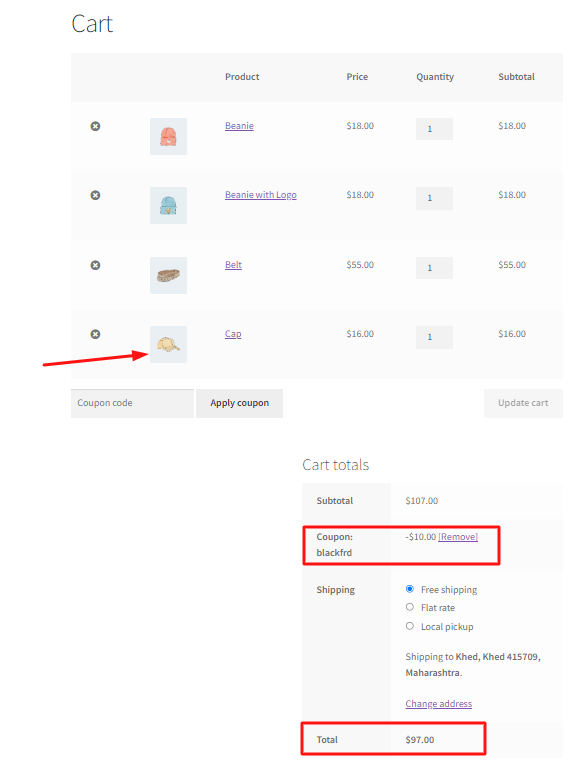
In another scenario where the cart has less than or equal to 3 items, the coupon code won’t apply as shown below.
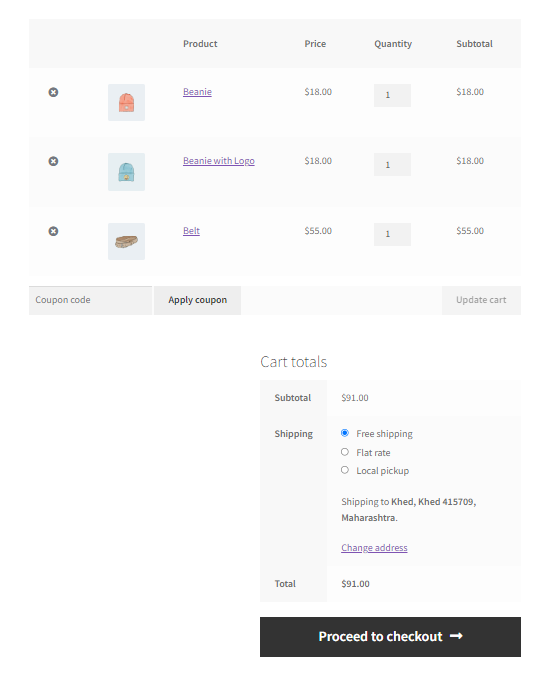
Code Explanation
The provided code snippets hook the function ts_apply_coupon_if_number_of_products to the woocommerce_before_cart action. This function checks the number of different products in the cart; if more than 3, it applies a coupon code (‘blackfrd’) if not already applied. If 3 or fewer products are present, it removes the coupon if applied. The code concludes by recalculating the cart totals to reflect the changes. This setup allows for dynamic application or removal of a coupon based on the number of products in the WooCommerce cart.
Conclusion
This code snippet enables automatic coupon application based on both cart total and specific product IDs. In this post, a generic coupon is being applied for all products to meet the required conditions. But instead of applying a generic coupon for all products you can also create unique coupon codes in WooCommerce programmatically.
Let us know your suggestions about the code in the comments below.
Hi prateek,
Thanks for the article, code snippets are also useful in this post.
I wanted to apply the coupon code when the number of products are added in the cart. I tired it, but couldn’t able to do so.
Can you please help me for this one?
Hi Kevin, The post has been updated as per your requirement. Please refer to the code snippets under the section ‘Solution 3: Apply coupon depending on the number of products in the cart.’