We all know how discounts lure customers and increase sales. But showing that you are selling an item for a lesser price isn’t sufficient these days, with all retailers & ecommerce websites offering discounts at the same time. When only the old and new prices are displayed, the customer is left to do the math to calculate how much amount he is actually saving. A better way to handle this is to display the percentage of the amount the customer saves when he makes that purchase. This gives them a better idea and an easier way to decide whether to make that purchase. Let’s see how you can display “You Save x%” next to sale prices for both simple and variable products in WooCommerce.
How to Display You Save % below sale prices for simple products
Displaying the percentage of amount saved is easy for simple products once the regular and the sale price is known. In normal cases, these prices for a simple product are displayed as follows:
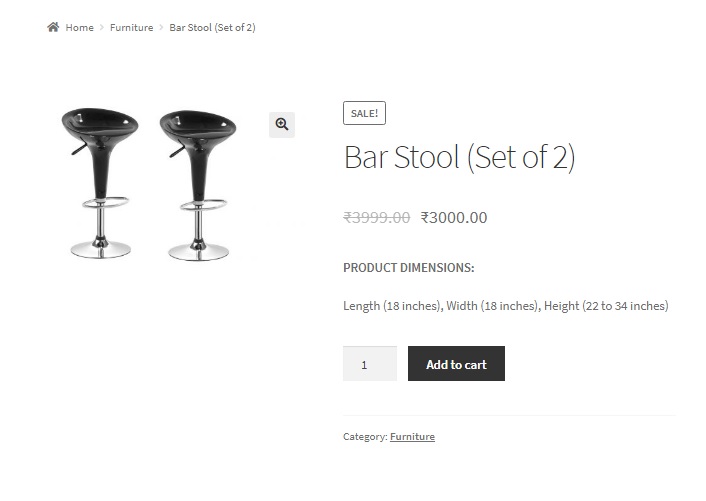
Adding the code below to the functions.php file of your child theme will display the “You Save x%” below the price of the simple product, so that the user immediately gets an idea of how good or bad the deal is.
function ts_you_save() { global $product; if( $product->is_type('simple') || $product->is_type('external') || $product->is_type('grouped') ) { $regular_price = get_post_meta( $product->get_id(), '_regular_price', true ); $sale_price = get_post_meta( $product->get_id(), '_sale_price', true ); if( !empty($sale_price) ) { $amount_saved = $regular_price - $sale_price; $currency_symbol = get_woocommerce_currency_symbol(); $percentage = round( ( ( $regular_price - $sale_price ) / $regular_price ) * 100 ); ?> <p style="font-size:24px;color:red;"><b>You Save: <?php echo number_format($percentage,0, '', '').'%'; ?></b></p> <?php } } } add_action( 'woocommerce_single_product_summary', 'ts_you_save', 11 );
In this code snippet, we have added a function ts_you_save() to the in-built WooCommerce hook woocommerce_single_product_summary, which points to the area below the product title. The function ts_you_save() identifies the type of the product and calculates the percentage of amount saved if the product is on sale. By adding some CSS, you can print this in the way you want to:
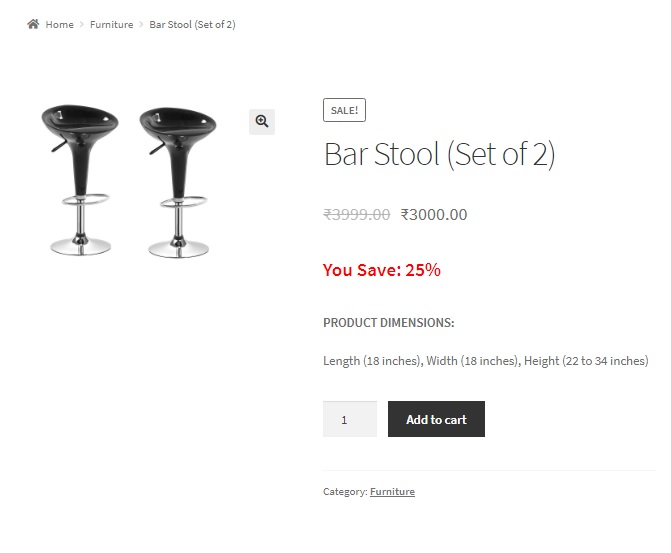
If you want to display the saved amount along with the percentage, you can modify the same code snippet just a little bit in the following way:
function ts_you_save() { global $product; if( $product->is_type('simple') || $product->is_type('external') || $product->is_type('grouped') ) { $regular_price = get_post_meta( $product->get_id(), '_regular_price', true ); $sale_price = get_post_meta( $product->get_id(), '_sale_price', true ); if( !empty($sale_price) ) { $amount_saved = $regular_price - $sale_price; $currency_symbol = get_woocommerce_currency_symbol(); $percentage = round( ( ( $regular_price - $sale_price ) / $regular_price ) * 100 ); ?> <p style="font-size:24px;color:red;"><b>You Save: <?php echo number_format($amount_saved,2, '.', '')." (". number_format($percentage,0, '', '')."%)"; ?></b></p> <?php } } } add_action( 'woocommerce_single_product_summary', 'ts_you_save', 11 );
The number_format function is an in-built PHP function which accepts 4 arguments:
- The number or in our case, the saved amount and percentage respectively (we have used the function twice).
- Number of decimal places. We have set it to 2 for the saved amount, and 0 for the percentage as we do not wish to display any decimal values for the percentage.
- The decimal separator you wish to use. We have set this to a dot(.) for the saved amount and a blank value for the percentage.
- The thousand separator. If the saved amount exceeds 1000, you can use this argument. We have set it to blank at present.
The saved amount as well as the percentage will now be displayed below the price:
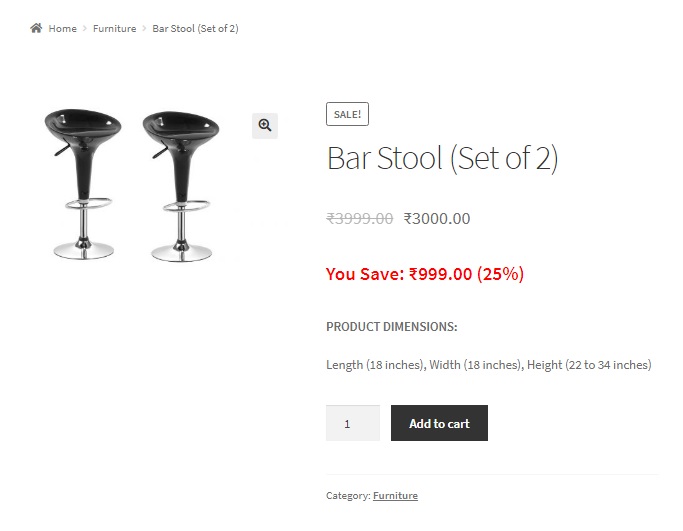
How to Display You Save % below sale prices for variable products
When it comes to variable products, this gets tricky because every variation of the product can have a different price. For example, a tshirt may be priced higher for a larger size while the price may be lower for the same tshirt in a smaller size. For cases like these, WooCommerce displays the sale price range below the product title, while the regular & sale prices of each variation of the product is displayed above the Add to Cart button when the variation is selected. This has been illustrated by the images below:
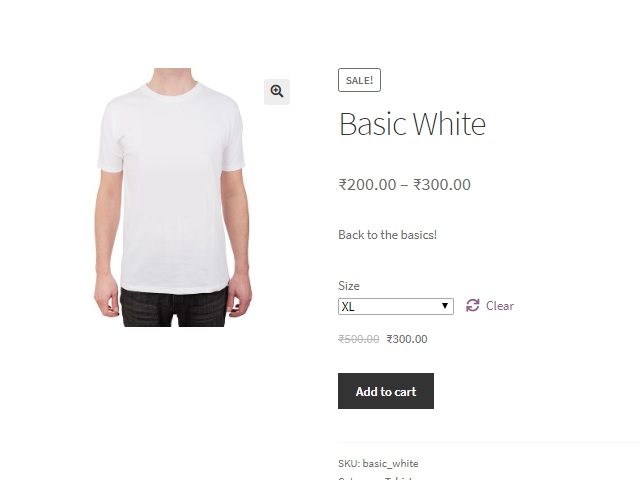
In this case, we will print the “You Save” text along with the saved percentage below the price that is displayed for the particular variation. You will need to add code snippets to both the functions.php and the footer.php files of your child theme.
The following code snippet that you will add in the footer.php file contains a function that is triggered when the variation is changed. In our case, this is triggered when the size variation is selected or changed using the Size dropdown. This function captures the particular variation id and sends it to functions.php via an ajax call.
<script> jQuery(document).ready(function($) { $('input.variation_id').change( function(){ if( '' != $('input.variation_id').val() ) { var var_id = $('input.variation_id').val(); // Your selected variation id $.ajax({ url: "https://<your_woocommerce_site_url>/wp-admin/admin-ajax.php", data: {'action':'ts_calc_percentage_saved','vari_id': var_id} , success: function(data) { if (data>0) { $( ".woocommerce-variation-price" ).append( "<p>You Save: "+data+"%</p>"); } }, error: function(errorThrown){ console.log(errorThrown); } }); } }); }); </script>
You need to replace <your_woocommerce_site_url> with the base URL of your WooCommerce website.
The following code snippet that you will add in the functions.php file contains a function ts_calc_percentage_saved that fetches the regular price & sale price of the product based on the variation id, calculates the percentage and returns the same to the ajax function in the footer.php file.
add_action("wp_ajax_nopriv_ts_calc_percentage_saved", "ts_calc_percentage_saved"); add_action("wp_ajax_ts_calc_percentage_saved", "ts_calc_percentage_saved"); // AJAX callback function function ts_calc_percentage_saved() { // Verify the AJAX request and user permissions if necessary if (isset($_REQUEST['vari_id'])) { $variation_id = absint($_REQUEST['vari_id']); $variable_product = wc_get_product($variation_id); if ($variable_product) { $regular_price = $variable_product->get_regular_price(); $sale_price = $variable_product->get_sale_price(); if (!empty($sale_price)) { $amount_saved = $regular_price - $sale_price; $percentage = round(($amount_saved / $regular_price) * 100); die(strval($percentage)); } } } // Handle any errors or invalid input die('0'); }
The sanitize_text_field() function is a WordPress function that helps to sanitize the input we receive from the client side.
Thus, you will now see “You Save”, along with the percentage of amount saved, below the price of the variation.
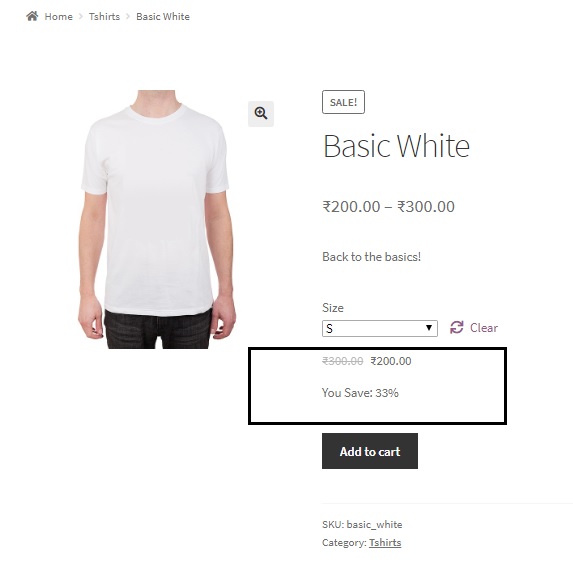
You can style this however you wish to, within the ajax function itself, by adding HTML and CSS within the append function on this line:
$( “.woocommerce-variation-price” ).append( “<p>You Save: “+data+”%</p>”);
Display You Save % below the product title instead of above the Add to Cart button for variable products
If you wish, you can display the You Save text along with the percentage saved below the product title instead of above the “Add to Cart” button by adding some more code snippets to the ones mentioned above.
This piece of code should be added to the functions.php file of your child theme, in addition to the code snippets mentioned above (in the footer.php and functions.php files).
//The below code is to shift the price function ts_shuffle_variable_product_elements(){ if ( is_product() ) { global $post; $product = wc_get_product( $post->ID ); if ( $product->is_type( 'variable' ) ) { remove_action( 'woocommerce_single_variation', 'woocommerce_single_variation', 10 ); add_action( 'woocommerce_before_variations_form', 'woocommerce_single_variation', 20 ); remove_action( 'woocommerce_single_product_summary', 'woocommerce_template_single_title', 5 ); add_action( 'woocommerce_before_variations_form', 'woocommerce_template_single_title', 10 ); remove_action( 'woocommerce_single_product_summary', 'woocommerce_template_single_excerpt', 20 ); add_action( 'woocommerce_before_variations_form', 'woocommerce_template_single_excerpt', 30 ); } } } add_action( 'woocommerce_before_single_product', 'ts_shuffle_variable_product_elements' );
What this code essentially does is remove the elements (including the title) and rearrange them the way you want to. The result of which is that the price range gets deleted and the variation price gets displayed when you select a variation, along with the You Save text below it, as illustrated:
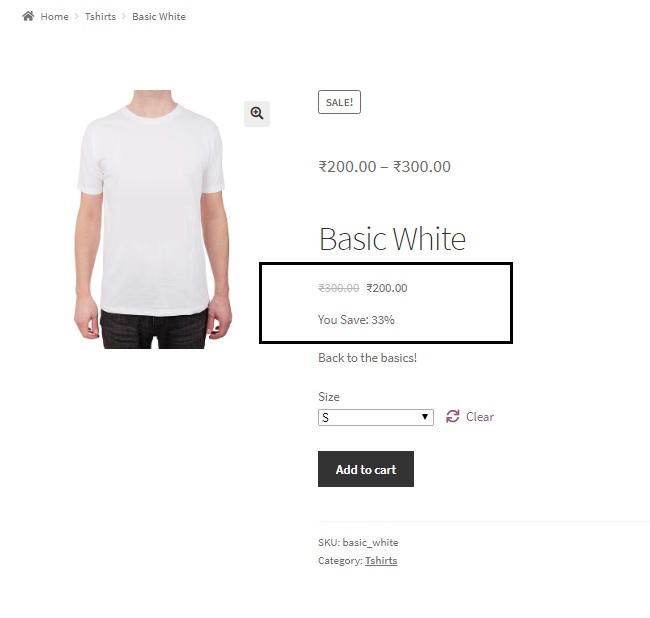
This code works great! The only problem I have is that on my bundled and single products, the saved amount and percentage always appears underneath the categories text right at the bottom of the page, which is a long way down for my bundled products. I want it to display right below the price. The code to add to the functions file and the footer file didn’t work for me (I’m not sure where to add in the footer file and if I need to add any other code around it for it to work).
Hi Helen, Glad to know that the code works well. The code snippets in this post uses two hooks, where one of them will place the ” You save: %” text just above the “Add to Cart ” button, and the hook in the last snippet will place the text immediately after the product title as shown here (https://prnt.sc/XkEioVVKKG8t). You can use any of these according to your needs. And regarding where to place the code, you should add the “jQuery script” code in the “footer.php” file and the “AJAX callback” code needs to be placed in “functions.php” of your child theme. To make sure that the implementation… Read more »
There’s something wrong when implementing the code for variable products. The You Save element is not displaying for mobile and tablet view. While it is working fine on desktop computers!
Hi, the code has been updated now. Please check the same.
Hi Guys. This does not work for me. I think it depends on your theme. Could u introduce a plugin for this situation instead of a code? thanks
Hi, the code has been updated. Please check the same.
This code is good for 2022?
And for variables produtcs it’s possible do insert “amount + percentage” for sales? How?
Hi, the code has been updated. Please check the same.
I’m trying to get the code for variable prices to work.
I have added the code to both footer.php and functions.php, and disabled all caching plugins just to be on the safe side.
Apparently the variable product dropdown still works, but the price disappears completely.
Using Chrome devtools reveals the all the HTML elements usually visible also disappear.
I’d appreciate any tips. I can forward a staging site address if needed-
Cheers, Kenny
Hi, the code has been updated. Please check the same.