Table of Contents
One of the core components of WooCommerce is the Order Object. The order object represents a single order placed by a customer on the online store. It contains valuable information about the order, including customer details, payment information, shipping address, order items, and more.
In this article, we will explore how to retrieve essential order information from the WooCommerce Order Object using built-in functions and custom code. Also, we will explore the process of accessing crucial order details including the order ID, order key, order totals, and order URLs.
Where to Add Custom Code in WooCommerce
It is advisable to add the code snippets to the functions.php file of your child theme. Access the file directly from Appearance->Theme File Editor->Locating the child theme’s functions.php from the right sidebar. You can also access it from your theme’s directory file. Insert the following code snippet in functions.php. The alternative & easy option is to install the Code Snippets plugin. You can then add the code as a new snippet via the plugin.
Getting the Order ID and Order Key
The first step in retrieving order information is obtaining the Order ID and Key. The Order ID is a unique identifier for each order, and the Order Key is a security token used for order-related actions. To get the Order ID and Order Key, you can use the following code:
// Assuming $order is the WooCommerce Order Object $order_id = $order->get_id(); $order_key = $order->get_order_key();
Get the Order Total Information
To access various order total information, such as parent ID, order version, order number, order date, formatted order total, cart tax, currency, discount tax, discount display, discount total, total fees, and more, you can use the following code:
// Get the order totals $parent_id = $order->get_parent_id(); $order_version = $order->get_version(); $order_number = $order->get_order_number(); $order_date = $order->get_date_created()->date_i18n('Y-m-d H:i:s'); $formatted_order_total = $order->get_formatted_order_total(); $cart_tax = $order->get_cart_tax(); $currency = $order->get_currency(); $discount_tax = $order->get_discount_tax(); $discount_display = $order->get_discount_to_display(); $discount_total = $order->get_discount_total(); $total_fees = $order->get_total_fees(); $shipping_tax = $order->get_shipping_tax(); $shipping_total = $order->get_shipping_total(); $get_subtotal = $subtotal = $order->get_subtotal(); $subtotal_to_display = $order->get_subtotal_to_display(); $get_tax_location = $order->get_tax_location(); $tax_totals = $order->get_tax_totals(); $taxes = $order->get_taxes(); $total = $order->get_total(); $total_discount= $order->get_total_discount(); $total_tax = $order->get_total_tax(); $total_refunded = $order->get_total_refunded(); $total_tax_refunded = $order->get_total_tax_refunded(); $total_shipping_refunded = $order->get_total_shipping_refunded(); $item_count_refunded = $order->get_item_count_refunded(); $total_qty_refunded = $order->get_total_qty_refunded(); $qty_refunded_for_item = $order->get_qty_refunded_for_item(); $total_refunded_for_item = $order->get_total_refunded_for_item(); $tax_refunded_for_item = $order->get_tax_refunded_for_item(); $total_tax_refunded_by_rate_id = $order->get_total_tax_refunded_by_rate_id(); $remaining_refund_amount = $order->get_remaining_refund_amount();
Get the Details of the Order dates
WooCommerce provides various dates associated with an order, such as date created, date modified, date completed, and date paid, along with their timestamps:
// Get the details of the Order Dates $date_created = $order->get_date_created()->date_i18n('Y-m-d H:i:s'); $date_modified = $order->get_date_modified()->date_i18n('Y-m-d H:i:s'); $date_completed = $order->get_date_completed() ? $order->get_date_completed()->date_i18n('Y-m-d H:i:s') : ''; $date_paid = $order->get_date_paid() ? $order->get_date_paid()->date_i18n('Y-m-d H:i:s') : ''; $timestamp_created = $order->get_date_created()->getTimestamp(); $timestamp_modified = $order->get_date_modified()->getTimestamp();
Get the Order Details for User & Customer
Retrieving user and customer information associated with the order can be done using the following code:
// Retrive the order details of the user and the customer $user_id = $order->get_user_id(); $customer_id = $order->get_customer_id(); $customer_email = $order->get_billing_email(); $customer_ip_address = $order->get_customer_ip_address(); $customer_user_agent = $order->get_customer_user_agent(); $customer_note = $order->get_customer_note();
Get the Order Shipping Address
To access the shipping address details associated with the order, use the following code:
// Retrive the details of the shipping address $shipping_first_name = $order->get_shipping_first_name(); $shipping_last_name = $order->get_shipping_last_name(); $shipping_company = $order->get_shipping_company(); $shipping_address_1 = $order->get_shipping_address_1(); $shipping_address_2 = $order->get_shipping_address_2(); $shipping_city = $order->get_shipping_city(); $shipping_postcode = $order->get_shipping_postcode(); $shipping_state = $order->get_shipping_state(); $shipping_country = $order->get_shipping_country(); $shipping_phone = $order->get_billing_phone(); $shipping_email = $order->get_billing_email(); // Formatted shipping full name and address $formatted_shipping_full_name = $order->get_formatted_shipping_full_name(); $formatted_shipping_address = $order->get_formatted_shipping_address();
Get the Order Billing Address
To retrieve billing address details, use the following code:
// Retrive the details of the order billing address $billing_first_name = $order->get_billing_first_name(); $billing_last_name = $order->get_billing_last_name(); $billing_company = $order->get_billing_company(); $billing_address_1 = $order->get_billing_address_1(); $billing_address_2 = $order->get_billing_address_2(); $billing_city = $order->get_billing_city(); $billing_postcode = $order->get_billing_postcode(); $billing_state = $order->get_billing_state(); $billing_country = $order->get_billing_country(); $billing_phone = $order->get_billing_phone(); $billing_email = $order->get_billing_email(); // Formatted billing full name and address $formatted_billing_full_name = $order->get_formatted_billing_full_name(); $formatted_billing_address = $order->get_formatted_billing_address();
Get the Order Payment Details
To access the payment method and payment method title used for the order, use the following code:
// Retrive the details of the order payments $payment_method = $order->get_payment_method(); //bacs $payment_method_title = $order->get_payment_method_title(); //Direct Bank Transfer
Here, is an output for the order payment method for Direct Bank Transfer for the above code as shown below.
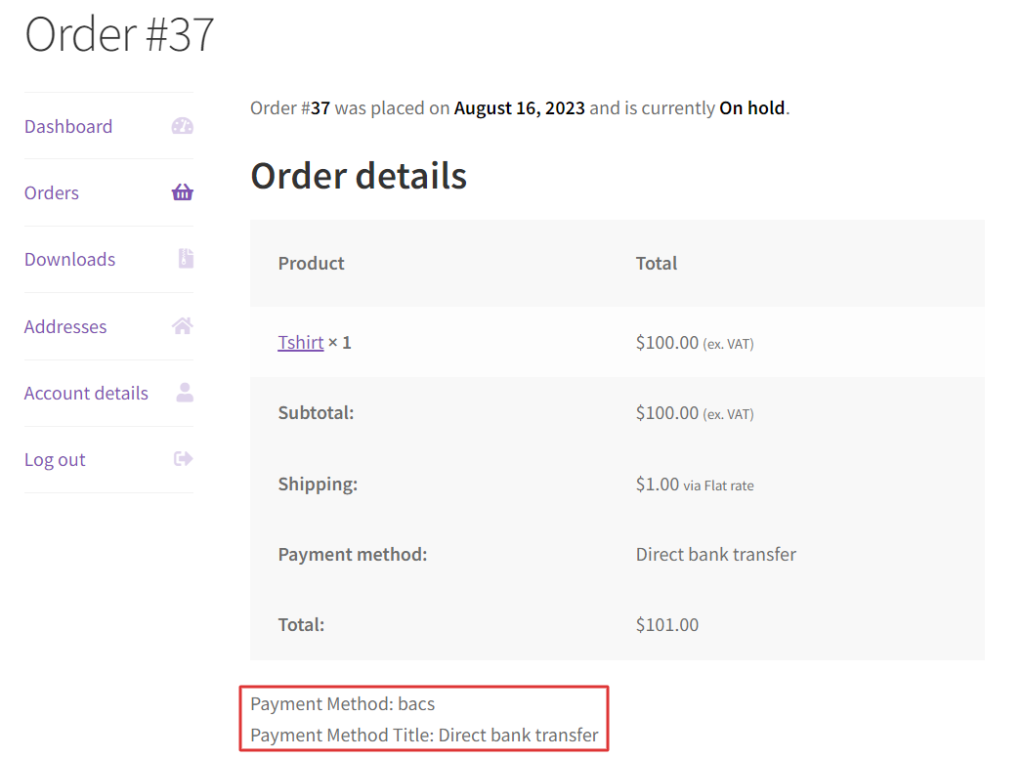
Get the Order URLs
WooCommerce provides several URLs associated with an order, making it easier for customers to navigate through the order process. To retrieve these URLs, use the following code:
// Retrive the details of the order URLs $checkout_payment_url = $order->get_checkout_payment_url(); $checkout_order_received_url = $order->get_checkout_order_received_url(); $cancel_order_url = $order->get_cancel_order_url(); $view_order_url = $order->get_view_order_url(); $edit_order_url = $order->get_edit_order_url();
Get the Order Status
The order status indicates the current state of the order such as Pending, Completed, On hold, etc. To access the order status, use the following code:
// Show the details of the order status $order_status = $order->get_status();
Get the Order Secondary Order Items
These secondary items are associated with an order like order items, downloadable items, and Coupon codes. You can access these details using the following code:
// Retrive the order secondary items $order_items = $order->get_items(); // Get items key and item tax classes $items_key = array_keys($order_items); $item_tax_classes = wp_list_pluck($order_items, 'tax_class'); // Get item count and downloadable items $item_count = count($order_items); $downloadable_items = $order->get_downloadable_items(); //these items are files that the customer can download after the purchase. // Get applied coupons $applied_coupons = $order->get_coupon_codes(); //This line fetches the applied coupon codes for the order.
Get Details About the Products in the Order
To access and extract product-specific information from the product object, such as product type, SKU, price, and quantity, and also access their details like product ID, name, variation ID, product details, line subtotal, line tax, line total, item name, quantity, and tax class use the following code:
// Retrive the data from the product object $order_items = $order->get_items(); foreach ($order_items as $item_id => $item_data) { // Get the product object $product = $item_data->get_product(); // Access product information $product_id = $item_data->get_product_id(); $product_type = $product->get_type(); $product_sku = $product->get_sku(); $product_price = $product->get_price(); $product_quantity = $item_data->get_quantity(); $variation_id = $item_data->get_variation_id(); $item_name = $item_data->get_name(); //Access individual Line Items $line_subtotal = $item_data->get_subtotal(); $line_tax = $item_data->get_subtotal_tax(); $line_total = $item_data->get_total(); $item_name = $item_data->get_name(); $item_quantity = $item_data->get_quantity(); $item_tax_class = $item_data->get_tax_class(); }
Retrieve $order object from other variables
Sometimes you may not have direct access to the $order object in the code. This issue may arise when working with hooks, filters, or custom functions where the $order object is not automatically passed as a parameter. In such cases, you may need to fetch the $order object from other variables or sources within their code.
Two common methods for retrieving the $order object involve using either the customer’s email or the order ID.
Access to $order_ID variable
The $order_ID variable is commonly used in WooCommerce to refer to the Order ID. Using this variable can simplify the retrieval of order information in your custom code.
// Get $order object from order ID $order = wc_get_order( $order_id ); if ( $order ) { $order->get_formatted_order_total( ); // etc. }
Access to $email variable
The $email variable is often used to refer to the customer’s email associated with the order. Utilizing this variable can make it easier to manage and use customer email information.
// Get $order object from $email $order = $email->object; if ( $order ) { $order->get_id(); $order->get_formatted_order_total( ); // etc. }
Conclusion
Using the $order object, you can able to perform the most advanced tasks like calculating order tax, generating invoices, sending the order confirmation email, and getting more information about the orders.
Hi prateek,
Article is good.
Can you please provide me the solution for retrieving the order id and key for every orders?