Table of Contents
We’ve all heard about the WordPress REST API now and then. The API allows us to access site data from other applications as needed. We can access WordPress data (using the existing API endpoints) as well as custom data from plugins etc. by creating custom API endpoints as needed.
Today, we’re going to look into creating a custom route and endpoint. This can essentially be helpful when we want plugin data to be accessed via the REST API. Before we dive in, we’ll first look at some basic concepts.
REST stands for Representational State Transfer. It is an architectural style that advocates that web applications should use HTTP.
The WordPress REST API provides API endpoints for WordPress data types that allow developers to interact with sites remotely by sending and receiving JSON (JavaScript Object Notation) objects. JSON is an open standard data format that is lightweight and human-readable, and looks like Objects do in JavaScript; hence the name.
When you send content to or make a request to the API, the response will be returned in JSON. This enables developers to create, read and update WordPress content from client-side JavaScript or from external applications, even those written in languages beyond PHP.
The WordPress REST API can be accessed at /wp-json/
. We can request access to the WordPress REST API index at https://mysite.com/wp-json/
. It lists basic site details like the site name, access URL, timezone, and API details like namespaces registered, endpoints available, and so on.
Some of the key concepts and terms that will help us get started with the API are as below:
- Routes/Endpoints
- Requests
- Responses
1. Routes & Endpoints
A route, in the context of the WordPress REST API, is a URI that can be mapped to different HTTP methods. The mapping of an individual HTTP method to a route is known as an endpoint. So essentially, endpoints are functions available through the API. These functions include things like updating a post, deleting a comment, and so on.
For e.g. wp/v2/posts/123
is a route. Here, wp/v2
is the namespace (explained in detail later), posts
indicates the type of data that is being retrieved and 123
is the post ID. This route has 3 endpoints
- GET triggers a
get_item
method, returning the post data to the client. - PUT triggers an
update_item
method, taking the data to update and returning the updated data to the client. - DELETE triggers the
delete_item
method, returning the now-deleted post data to the client.
2. Requests
One of the primary classes in the WordPress REST API infrastructure is
WP_REST_Request. This class is used to store and retrieve information for the current request. Requests can be submitted remotely via HTTP but may also be made internally from PHP with WordPress.
3. Responses
Responses are the data you get back from the API. The
WP_REST_Response class provides a way to interact with the response data returned by endpoints. Responses can return the desired data, and they can also be used to return errors.
WordPress has routes and endpoints to read, update, delete almost all of its data. However, since there are thousands of plugins based on WordPress out there, it is safe to assume that those plugins will have their custom data, which they will need access to using the WordPress REST API.
Rest easy, WordPress allows us to create our own custom routes and endpoints. WordPress provides us with hooks and functions to do the needful. Taking an example, we will write a patch of code to register a new route that allows us to return some simple text.
Creating a custom endpoint
As the name suggests,
register_rest_route is the function that we need to use to register a new route with WordPress. This function needs to be added in a function attached to the
rest_api_init hook.
The first argument in the register_rest_route
function is the namespace. This namespace helps us to group together all our routes.
It is extremely important to add namespaces to your routes. The “core” endpoints use the wp/v2
namespace. The first part of the namespace is wp
, which represents the vendor name; WordPress and v2 is the version number. We’re going to use my-route
as the namespace here.
The second argument is the resource path. The resource path signifies what resource the endpoint is associated with (like posts, comments and so on). In our case, we will name it my-phrase.
The third argument is an array of options. Here we specify what methods the endpoint can use and callback functions for each method. This argument also allows us to provide a permissions callback, which can restrict access for the endpoint to certain users.
Once this endpoint is created, you can access it at the below url https://mysite.com/wp-json/my-route/my-phrase
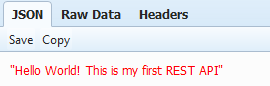
rest_ensure_response is the function that is used to return the data. This function returns a new instance of the WP_REST_RESPONSE
class for the data being returned. This is needed to make sure the data is returned in the correct format. The function also allows for errors to be passed back.
Restricting access to the endpoint
Restricting access can be achieved by using 'permission_callback'
.
The permission_callback
argument allows us to setup permissions making sure the data is accessed only by users who have authority to access it.
In the example above, I’ve limited the access to only those users that have the 'edit_posts'
authority. So if I access the link https://mysite.com/wp-json/my-route/my-phrase
as a guest or a logged in user with authority lower than that of an author, I will see an error message as below:
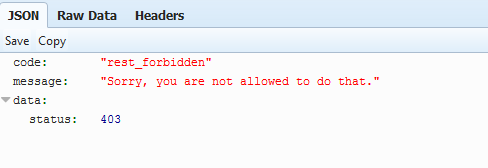
We will now look at an example to fetch some post data. Although this example simply fetches some data for all the posts on the site, you can write the code to fetch your custom plugin data on similar lines.
Fetching WordPress data using an endpoint
The above code is very similar to what we have done in our first example in this post.
We first registered an endpoint as my-posts
. In the callback function, we fetched all the posts and returned some data from the same.
wp_reset_postdata function ensures that the global $post
is restored to the current post in the main query. get_posts()
uses WP_Query
, hence it is necessary to ensure we restore the post data.
You can access the results from the above code on https://mysite.com/wp-json/my-route/my-posts/
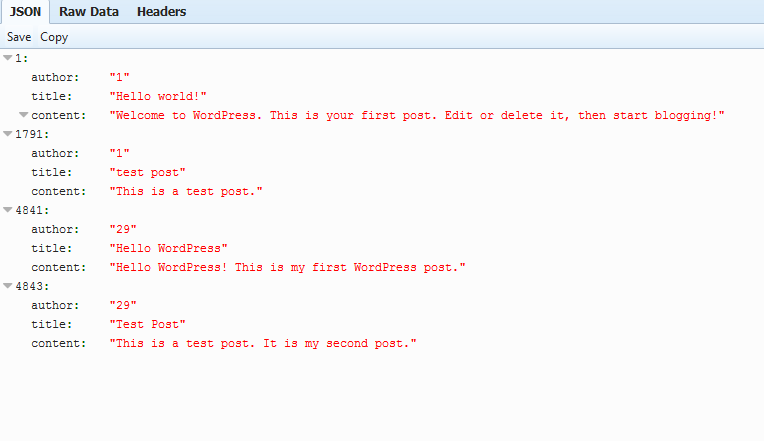
The above is a list of all the posts present on the site irrespective of the author.
Filtering the data
Continuing on the above example, we will now try to fetch posts for a given author only.
We have added a new argument in the third parameter being passed to the register_rest_route
function. 'args'
allows us to add as many arguments as we want. Based on the same, the data will be filtered. Note that we’ve also modified the route to ensure that the author ID is an expected parameter.
As you might’ve noticed in the first screenshot of the posts, we have 2 posts each from 2 different authors.
When we access the path https://mysite.com/wp-json/my-route/my-posts/1
it will display the posts with the author ID 1.
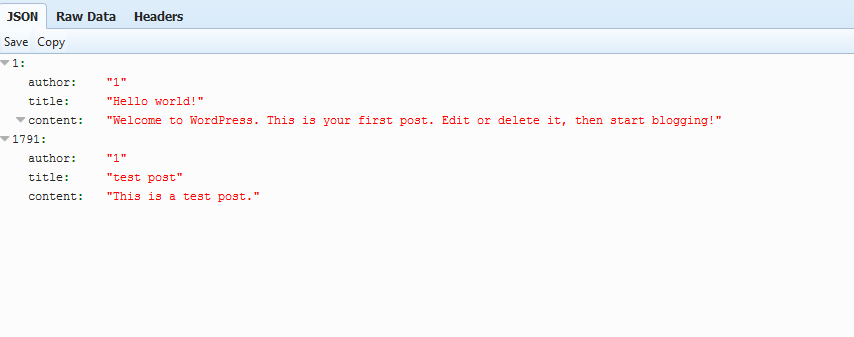
Similarly, when we access the path https://mysite.com/wp-json/my-route/my-posts/29
, it will display the posts from the author ID 29.
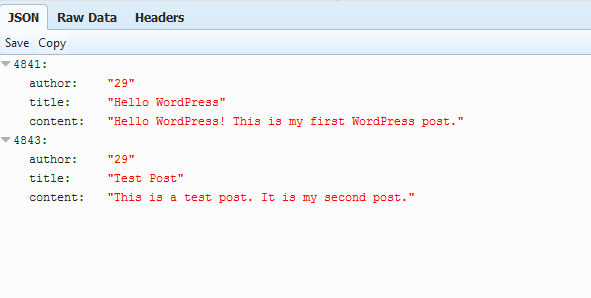
Being able to read data from a WordPress site using the REST API is just skimming the surface of the pond. The API allows you to not only read the data, it enables you to filter the data based on the arguments passed, validate that the user has the authority to view the data as well.
Apart from that, you can edit, create and delete data using the API as well.
I hope this post helps you get started with creating your custom API endpoints. To help you move forward with this, the WordPress REST API handbook is located here. In case if you have tried using the same methods or alternate methods with better results, please let us know in the comments below!
how to get post revisions as a query string?
I have wrote an endpoint in WordPress and it works just fine. The problem is with the URL. The current format of the URL request must be like this:
https://iotkidsiq.com/wp-json/zaindob/v1/sync_order/key=011900/msisdn=019009
However, The URL request must be in this format:
https://iotkidsiq.com/wp-json/zaindob/v1/sync_order?key=011900&msisdn=019009
How to format that?
WordPress 4.9.8 why show this error ?
{
“code”: “rest_forbidden”,
“message”: “Sorry, you are not allowed to do that.”,
“data”: {
“status”: 401
}
}
adding the permission callback require you to pass basic auth param like username and password for user who can do, from the example above, ‘edit_post’. Anyways, thank you for the author for this clear and brief explanation! Thumbs up!